Track notebooks, scripts & functions¶
This guide explains how to use track()
& finish()
to track notebook & scripts along with their inputs and outputs.
For tracking pipelines, see: Pipelines – workflow managers.
# !pip install 'lamindb[jupyter]'
!lamin init --storage ./test-track
Show code cell output
→ initialized lamindb: testuser1/test-track
Track a notebook or script¶
Call track()
to register your notebook or script as a transform
and start tracking inputs & outputs of a run.
import lamindb as ln
ln.track() # initiate a tracked notebook/script run
# your code
ln.finish() # mark run as finished, save execution report, source code & environment
Here is how a notebook with run report looks on the hub.
Explore it here.
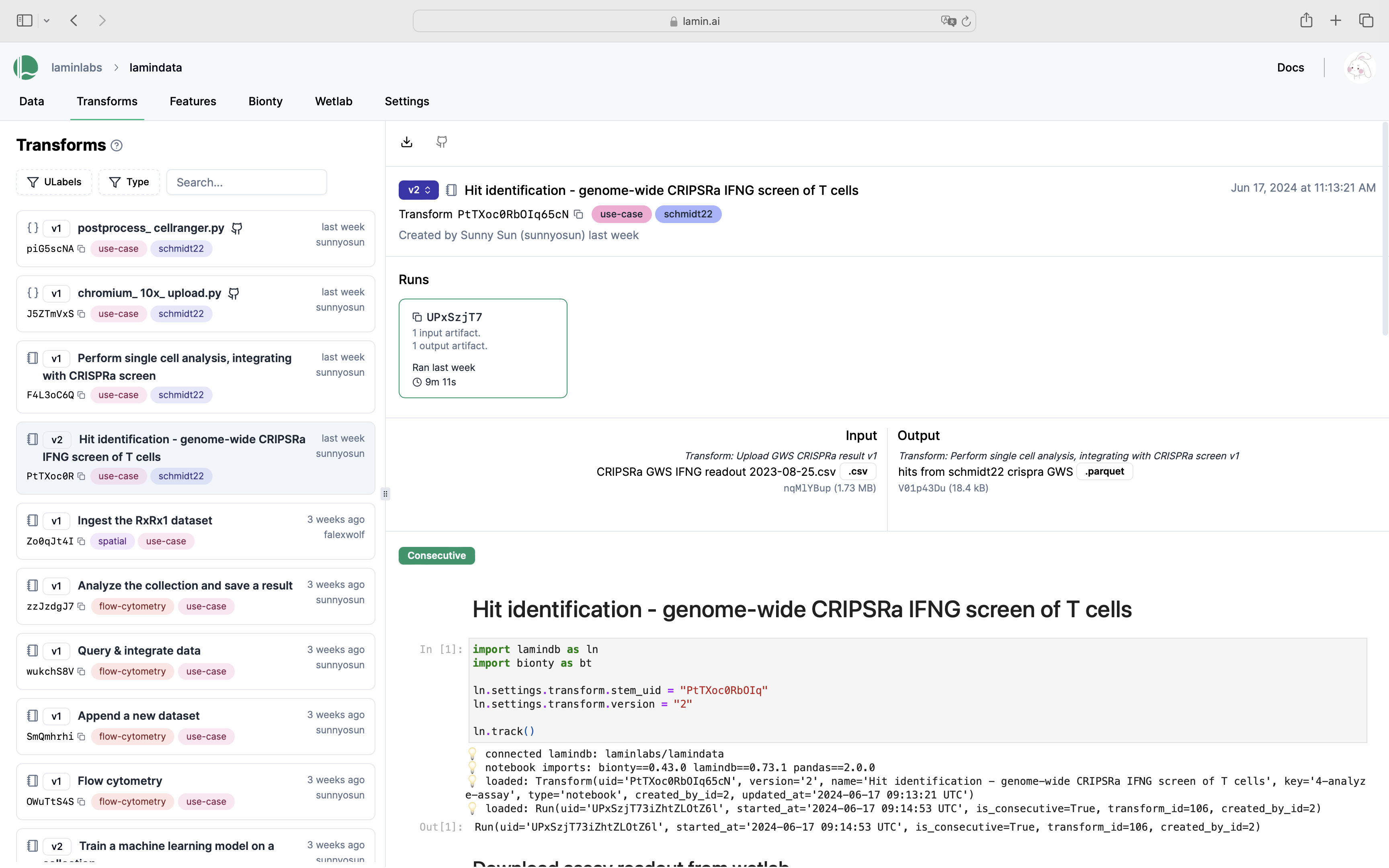
Assign a uid
to a notebook or script
If you want to retain one version history when renaming notebooks and scripts, pass a uid
to ln.track()
, e.g.
ln.track("9priar0hoE5u0000")
To obtain a uid
value, copy it from the logging statement (Transform('9priar0hoE5u0000')
) when running ln.track()
a first time without passing a uid
.
Load a notebook or script¶
On the hub, search or filter the transform
page and then load a script or notebook on the CLI. For example,
lamin load https://lamin.ai/laminlabs/lamindata/transform/13VINnFk89PE
Query a notebook or script¶
You find your notebooks and scripts in the Transform
registry (along with pipelines & functions). Run
stores executions.
You can use all usual ways of querying to obtain one or multiple transform records, e.g.:
transform = ln.Transform.get(key="my_analyses/my_notebook.ipynb")
transform.source_code # source code
transform.latest_run.report # report of latest run
transform.latest_run.environment # environment of latest run
transform.runs # all runs
Sync scripts with git¶
To sync with your git commit, add the following line to your script:
ln.settings.sync_git_repo = <YOUR-GIT-REPO-URL>
import lamindb as ln
ln.settings.sync_git_repo = "https://github.com/..."
ln.track()
# your code
ln.finish()
You’ll now see the GitHub emoji clickable on the hub.

Track parameters¶
In addition to tracking source code, run reports & environments, you can easily track run parameters.
Track run parameters¶
Before tracking parameter values, you need to define valid parameters, e.g.:
import lamindb as ln
ln.Param(name="input_dir", dtype="str").save()
ln.Param(name="learning_rate", dtype="float").save()
ln.Param(name="preprocess_params", dtype="dict").save()
Show code cell output
→ connected lamindb: testuser1/test-track
Param(name='preprocess_params', dtype='dict', created_by_id=1, space_id=1, created_at=2025-02-18 13:41:10 UTC)
Upon running the below script without those parameters defined, you’ll get a ValidationError
from which you can copy & paste the definitions.
import argparse
import lamindb as ln
if __name__ == "__main__":
p = argparse.ArgumentParser()
p.add_argument("--input-dir", type=str)
p.add_argument("--downsample", action="store_true")
p.add_argument("--learning-rate", type=float)
args = p.parse_args()
params = {
"input_dir": args.input_dir,
"learning_rate": args.learning_rate,
"preprocess_params": {
"downsample": args.downsample, # nested parameter names & values in dictionaries are not validated
"normalization": "the_good_one",
},
}
ln.track(params=params)
# your code
ln.finish()
Run the script.
!python scripts/run-track-with-params.py --input-dir ./mydataset --learning-rate 0.01 --downsample
Show code cell output
→ connected lamindb: testuser1/test-track
→ created Transform('j7Ecw9WoDmgs0000'), started new Run('Qvbucg6h...') at 2025-02-18 13:41:14 UTC
→ params: input_dir=./mydataset, learning_rate=0.01, preprocess_params={'downsample': True, 'normalization': 'the_good_one'}
→ finished Run('Qvbucg6h') after 2s at 2025-02-18 13:41:16 UTC
Query by run parameters¶
Query for all runs that match a certain parameters:
ln.Run.params.filter(
learning_rate=0.01, input_dir="./mydataset", preprocess_params__downsample=True
).df()
Show code cell output
uid | name | started_at | finished_at | reference | reference_type | _is_consecutive | _status_code | space_id | transform_id | report_id | _logfile_id | environment_id | initiated_by_run_id | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
id | ||||||||||||||||||
1 | Qvbucg6hc7fSfyQp0aK2 | None | 2025-02-18 13:41:14.027840+00:00 | 2025-02-18 13:41:16.065501+00:00 | None | None | True | 0 | 1 | 1 | 2 | None | 1 | None | 2025-02-18 13:41:14.028000+00:00 | 1 | None | 1 |
Note that:
preprocess_params__downsample=True
traverses the dictionarypreprocess_params
to find the key"downsample"
and match it toTrue
nested keys like
"downsample"
in a dictionary do not appear inParam
and hence, do not get validated
Access parameters of a run¶
Below is how you get the parameter values that were used for a given run.
run = ln.Run.params.filter(learning_rate=0.01).order_by("-started_at").first()
run.params.get_values()
Show code cell output
{'input_dir': './mydataset',
'learning_rate': 0.01,
'preprocess_params': {'downsample': True, 'normalization': 'the_good_one'}}
Here is how it looks on the hub.
Explore all parameter values¶
If you want to query all parameter values across all runs, use ParamValue
.
ln.core.ParamValue.df(include=["param__name", "created_by__handle"])
Show code cell output
value | hash | space_id | param__name | created_by__handle | |
---|---|---|---|---|---|
id | |||||
1 | ./mydataset | None | 1 | input_dir | testuser1 |
2 | 0.01 | None | 1 | learning_rate | testuser1 |
3 | {'downsample': True, 'normalization': 'the_goo... | None | 1 | preprocess_params | testuser1 |
Show code cell content
assert run.params.get_values() == {
"input_dir": "./mydataset",
"learning_rate": 0.01,
"preprocess_params": {"downsample": True, "normalization": "the_good_one"},
}
Manage notebook templates¶
A notebook acts like a template upon using lamin load
to load it. Consider you run:
lamin load https://lamin.ai/account/instance/transform/Akd7gx7Y9oVO0000
Upon running the returned notebook, you’ll automatically create a new version and be able to browse it via the version dropdown on the UI.
Additionally, you can:
label using
ULabel
, e.g.,transform.ulabels.add(template_label)
tag with an indicative
version
string, e.g.,transform.version = "T1"; transform.save()
Saving a notebook as an artifact
Sometimes you might want to save a notebook as an artifact. This is how you can do it:
lamin save template1.ipynb --key templates/template1.ipynb --description "Template for analysis type 1" --registry artifact
Track functions¶
If you want more-fined-grained data lineage tracking, use the tracked()
decorator.
In a notebook¶
import lamindb as ln
ln.track() # track this notebook
ln.Param(name="subset_rows", dtype="int").save() # define parameters
ln.Param(name="subset_cols", dtype="int").save()
ln.Param(name="input_artifact_key", dtype="str").save()
ln.Param(name="output_artifact_key", dtype="str").save()
→ created Transform('Hgy4VKDv3anM0000'), started new Run('lBVxeHIL...') at 2025-02-18 13:41:18 UTC
→ notebook imports: lamindb==1.1.0
Param(name='output_artifact_key', dtype='str', created_by_id=1, run_id=2, space_id=1, created_at=2025-02-18 13:41:19 UTC)
Define a function and decorate it with tracked()
:
@ln.tracked()
def subset_dataframe(
input_artifact_key: str,
output_artifact_key: str,
subset_rows: int = 2,
subset_cols: int = 2,
) -> None:
artifact = ln.Artifact.get(key=input_artifact_key)
dataset = artifact.load()
new_data = dataset.iloc[:subset_rows, :subset_cols]
ln.Artifact.from_df(new_data, key=output_artifact_key).save()
Prepare a test dataset:
df = ln.core.datasets.small_dataset1(otype="DataFrame")
input_artifact_key = "my_analysis/dataset.parquet"
artifact = ln.Artifact.from_df(df, key=input_artifact_key).save()
Run the function with default params:
ouput_artifact_key = input_artifact_key.replace(".parquet", "_subsetted.parquet")
subset_dataframe(input_artifact_key, ouput_artifact_key)
Query for the output:
subsetted_artifact = ln.Artifact.get(key=ouput_artifact_key)
subsetted_artifact.view_lineage()
This is the run that created the subsetted_artifact:
subsetted_artifact.run
Run(uid='K0mfvaqZti09j1AaJq44', started_at=2025-02-18 13:41:19 UTC, finished_at=2025-02-18 13:41:19 UTC, space_id=1, transform_id=3, created_by_id=1, initiated_by_run_id=2, created_at=2025-02-18 13:41:19 UTC)
This is the function that created it:
subsetted_artifact.run.transform
Transform(uid='r0W2E5c3booT0000', is_latest=True, key='track.ipynb/subset_dataframe.py', type='function', hash='F_wwrfFs6zmzMGVilG2Prg', space_id=1, created_by_id=1, created_at=2025-02-18 13:41:19 UTC)
This is the source code of this function:
subsetted_artifact.run.transform.source_code
'@ln.tracked()\ndef subset_dataframe(\n input_artifact_key: str,\n output_artifact_key: str,\n subset_rows: int = 2,\n subset_cols: int = 2,\n) -> None:\n artifact = ln.Artifact.get(key=input_artifact_key)\n dataset = artifact.load()\n new_data = dataset.iloc[:subset_rows, :subset_cols]\n ln.Artifact.from_df(new_data, key=output_artifact_key).save()\n'
These are all versions of this function:
subsetted_artifact.run.transform.versions.df()
uid | key | description | type | source_code | hash | reference | reference_type | space_id | _template_id | version | is_latest | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
id | ||||||||||||||||
3 | r0W2E5c3booT0000 | track.ipynb/subset_dataframe.py | None | function | @ln.tracked()\ndef subset_dataframe(\n inpu... | F_wwrfFs6zmzMGVilG2Prg | None | None | 1 | None | None | True | 2025-02-18 13:41:19.848000+00:00 | 1 | None | 1 |
This is the initating run that triggered the function call:
subsetted_artifact.run.initiated_by_run
Run(uid='lBVxeHILVLR7iB0vwCDL', started_at=2025-02-18 13:41:18 UTC, space_id=1, transform_id=2, created_by_id=1, created_at=2025-02-18 13:41:18 UTC)
This is the transform
of the initiating run:
subsetted_artifact.run.initiated_by_run.transform
Transform(uid='Hgy4VKDv3anM0000', is_latest=True, key='track.ipynb', description='Track notebooks, scripts & functions', type='notebook', space_id=1, created_by_id=1, created_at=2025-02-18 13:41:18 UTC)
These are the parameters of the run:
subsetted_artifact.run.params.get_values()
{'input_artifact_key': 'my_analysis/dataset.parquet',
'output_artifact_key': 'my_analysis/dataset_subsetted.parquet',
'subset_cols': 2,
'subset_rows': 2}
These input artifacts:
subsetted_artifact.run.input_artifacts.df()
uid | key | description | suffix | kind | otype | size | hash | n_files | n_observations | _hash_type | _key_is_virtual | _overwrite_versions | space_id | storage_id | schema_id | version | is_latest | run_id | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
id | |||||||||||||||||||||||
3 | PhKAkwD9McPMysfR0000 | my_analysis/dataset.parquet | None | .parquet | dataset | DataFrame | 6586 | yMhflyJSY9zEHe9ew5-JqA | None | None | md5 | True | False | 1 | 1 | None | None | True | 2 | 2025-02-18 13:41:19.827000+00:00 | 1 | None | 1 |
These are output artifacts:
subsetted_artifact.run.output_artifacts.df()
uid | key | description | suffix | kind | otype | size | hash | n_files | n_observations | _hash_type | _key_is_virtual | _overwrite_versions | space_id | storage_id | schema_id | version | is_latest | run_id | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
id | |||||||||||||||||||||||
4 | GGCIFFTYUfmJn5JS0000 | my_analysis/dataset_subsetted.parquet | None | .parquet | dataset | DataFrame | 3233 | 7jKFlRPwu3gPRxVnkb6qaw | None | None | md5 | True | False | 1 | 1 | None | None | True | 3 | 2025-02-18 13:41:19.901000+00:00 | 1 | None | 1 |
Re-run the function with a different parameter:
subsetted_artifact = subset_dataframe(
input_artifact_key, ouput_artifact_key, subset_cols=3
)
subsetted_artifact = ln.Artifact.get(key=ouput_artifact_key)
subsetted_artifact.view_lineage()
Show code cell output
→ creating new artifact version for key='my_analysis/dataset_subsetted.parquet' (storage: '/home/runner/work/lamindb/lamindb/docs/test-track')
We created a new run:
subsetted_artifact.run
Run(uid='ayroJ8z2cuoa8xEZsbgM', started_at=2025-02-18 13:41:20 UTC, finished_at=2025-02-18 13:41:20 UTC, space_id=1, transform_id=3, created_by_id=1, initiated_by_run_id=2, created_at=2025-02-18 13:41:20 UTC)
With new parameters:
subsetted_artifact.run.params.get_values()
{'input_artifact_key': 'my_analysis/dataset.parquet',
'output_artifact_key': 'my_analysis/dataset_subsetted.parquet',
'subset_cols': 3,
'subset_rows': 2}
And a new version of the output artifact:
subsetted_artifact.run.output_artifacts.df()
uid | key | description | suffix | kind | otype | size | hash | n_files | n_observations | _hash_type | _key_is_virtual | _overwrite_versions | space_id | storage_id | schema_id | version | is_latest | run_id | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
id | |||||||||||||||||||||||
5 | GGCIFFTYUfmJn5JS0001 | my_analysis/dataset_subsetted.parquet | None | .parquet | dataset | DataFrame | 3839 | s5a7sMZMWEXie9Oy-UDK5g | None | None | md5 | True | False | 1 | 1 | None | None | True | 4 | 2025-02-18 13:41:20.424000+00:00 | 1 | None | 1 |
See the state of the database:
ln.view()
Show code cell output
Artifact
uid | key | description | suffix | kind | otype | size | hash | n_files | n_observations | _hash_type | _key_is_virtual | _overwrite_versions | space_id | storage_id | schema_id | version | is_latest | run_id | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
id | |||||||||||||||||||||||
5 | GGCIFFTYUfmJn5JS0001 | my_analysis/dataset_subsetted.parquet | None | .parquet | dataset | DataFrame | 3839 | s5a7sMZMWEXie9Oy-UDK5g | None | None | md5 | True | False | 1 | 1 | None | None | True | 4 | 2025-02-18 13:41:20.424000+00:00 | 1 | None | 1 |
4 | GGCIFFTYUfmJn5JS0000 | my_analysis/dataset_subsetted.parquet | None | .parquet | dataset | DataFrame | 3233 | 7jKFlRPwu3gPRxVnkb6qaw | None | None | md5 | True | False | 1 | 1 | None | None | False | 3 | 2025-02-18 13:41:19.901000+00:00 | 1 | None | 1 |
3 | PhKAkwD9McPMysfR0000 | my_analysis/dataset.parquet | None | .parquet | dataset | DataFrame | 6586 | yMhflyJSY9zEHe9ew5-JqA | None | None | md5 | True | False | 1 | 1 | None | None | True | 2 | 2025-02-18 13:41:19.827000+00:00 | 1 | None | 1 |
Param
name | dtype | is_type | _expect_many | space_id | type_id | run_id | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|---|---|---|
id | |||||||||||
7 | output_artifact_key | str | None | False | 1 | None | 2.0 | 2025-02-18 13:41:19.763000+00:00 | 1 | None | 1 |
6 | input_artifact_key | str | None | False | 1 | None | 2.0 | 2025-02-18 13:41:19.756000+00:00 | 1 | None | 1 |
5 | subset_cols | int | None | False | 1 | None | 2.0 | 2025-02-18 13:41:19.749000+00:00 | 1 | None | 1 |
4 | subset_rows | int | None | False | 1 | None | 2.0 | 2025-02-18 13:41:19.742000+00:00 | 1 | None | 1 |
3 | preprocess_params | dict | None | False | 1 | None | NaN | 2025-02-18 13:41:10.129000+00:00 | 1 | None | 1 |
2 | learning_rate | float | None | False | 1 | None | NaN | 2025-02-18 13:41:10.123000+00:00 | 1 | None | 1 |
1 | input_dir | str | None | False | 1 | None | NaN | 2025-02-18 13:41:10.111000+00:00 | 1 | None | 1 |
ParamValue
value | hash | space_id | param_id | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|
id | ||||||||
1 | ./mydataset | None | 1 | 1 | 2025-02-18 13:41:14.048000+00:00 | 1 | None | 1 |
2 | 0.01 | None | 1 | 2 | 2025-02-18 13:41:14.048000+00:00 | 1 | None | 1 |
3 | {'downsample': True, 'normalization': 'the_goo... | None | 1 | 3 | 2025-02-18 13:41:14.048000+00:00 | 1 | None | 1 |
4 | my_analysis/dataset.parquet | None | 1 | 6 | 2025-02-18 13:41:19.872000+00:00 | 1 | None | 1 |
5 | my_analysis/dataset_subsetted.parquet | None | 1 | 7 | 2025-02-18 13:41:19.872000+00:00 | 1 | None | 1 |
6 | 2 | None | 1 | 4 | 2025-02-18 13:41:19.872000+00:00 | 1 | None | 1 |
7 | 2 | None | 1 | 5 | 2025-02-18 13:41:19.872000+00:00 | 1 | None | 1 |
Run
uid | name | started_at | finished_at | reference | reference_type | _is_consecutive | _status_code | space_id | transform_id | report_id | _logfile_id | environment_id | initiated_by_run_id | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
id | ||||||||||||||||||
1 | Qvbucg6hc7fSfyQp0aK2 | None | 2025-02-18 13:41:14.027840+00:00 | 2025-02-18 13:41:16.065501+00:00 | None | None | True | 0 | 1 | 1 | 2.0 | None | 1.0 | NaN | 2025-02-18 13:41:14.028000+00:00 | 1 | None | 1 |
2 | lBVxeHILVLR7iB0vwCDL | None | 2025-02-18 13:41:18.252149+00:00 | NaT | None | None | None | 0 | 1 | 2 | NaN | None | NaN | NaN | 2025-02-18 13:41:18.253000+00:00 | 1 | None | 1 |
3 | K0mfvaqZti09j1AaJq44 | None | 2025-02-18 13:41:19.852146+00:00 | 2025-02-18 13:41:19.904506+00:00 | None | None | None | 0 | 1 | 3 | NaN | None | NaN | 2.0 | 2025-02-18 13:41:19.852000+00:00 | 1 | None | 1 |
4 | ayroJ8z2cuoa8xEZsbgM | None | 2025-02-18 13:41:20.364973+00:00 | 2025-02-18 13:41:20.429714+00:00 | None | None | None | 0 | 1 | 3 | NaN | None | NaN | 2.0 | 2025-02-18 13:41:20.365000+00:00 | 1 | None | 1 |
Storage
uid | root | description | type | region | instance_uid | space_id | run_id | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|---|---|---|---|
id | ||||||||||||
1 | CPhhQrlufozK | /home/runner/work/lamindb/lamindb/docs/test-track | None | local | None | 73KPGC58ahU9 | 1 | None | 2025-02-18 13:41:04.256000+00:00 | 1 | None | 1 |
Transform
uid | key | description | type | source_code | hash | reference | reference_type | space_id | _template_id | version | is_latest | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
id | ||||||||||||||||
3 | r0W2E5c3booT0000 | track.ipynb/subset_dataframe.py | None | function | @ln.tracked()\ndef subset_dataframe(\n inpu... | F_wwrfFs6zmzMGVilG2Prg | None | None | 1 | None | None | True | 2025-02-18 13:41:19.848000+00:00 | 1 | None | 1 |
2 | Hgy4VKDv3anM0000 | track.ipynb | Track notebooks, scripts & functions | notebook | None | None | None | None | 1 | None | None | True | 2025-02-18 13:41:18.245000+00:00 | 1 | None | 1 |
1 | j7Ecw9WoDmgs0000 | run-track-with-params.py | run-track-with-params.py | script | import argparse\nimport lamindb as ln\n\nif __... | nRUs3ZjuVTbKtBmSXpVQ5A | None | None | 1 | None | None | True | 2025-02-18 13:41:14.024000+00:00 | 1 | None | 1 |
In a script¶
import argparse
import lamindb as ln
ln.Param(name="run_workflow_subset", dtype=bool).save()
@ln.tracked()
def subset_dataframe(
artifact: ln.Artifact,
subset_rows: int = 2,
subset_cols: int = 2,
run: ln.Run | None = None,
) -> ln.Artifact:
dataset = artifact.load(is_run_input=run)
new_data = dataset.iloc[:subset_rows, :subset_cols]
new_key = artifact.key.replace(".parquet", "_subsetted.parquet")
return ln.Artifact.from_df(new_data, key=new_key, run=run).save()
if __name__ == "__main__":
p = argparse.ArgumentParser()
p.add_argument("--subset", action="store_true")
args = p.parse_args()
params = {"run_workflow_subset": args.subset}
ln.track(params=params)
if args.subset:
df = ln.core.datasets.small_dataset1(otype="DataFrame")
artifact = ln.Artifact.from_df(df, key="my_analysis/dataset.parquet").save()
subsetted_artifact = subset_dataframe(artifact)
ln.finish()
!python scripts/run-workflow.py --subset
Show code cell output
→ connected lamindb: testuser1/test-track
→ created Transform('US4rlnbl6eKX0000'), started new Run('uiMoQAnZ...') at 2025-02-18 13:41:24 UTC
→ params: run_workflow_subset=True
→ found artifact with same hash: Artifact(uid='PhKAkwD9McPMysfR0000', is_latest=True, key='my_analysis/dataset.parquet', suffix='.parquet', kind='dataset', otype='DataFrame', size=6586, hash='yMhflyJSY9zEHe9ew5-JqA', space_id=1, storage_id=1, run_id=2, created_by_id=1, created_at=2025-02-18 13:41:19 UTC); to track this artifact as an input, use: ln.Artifact.get()
→ found artifact with same hash: Artifact(uid='GGCIFFTYUfmJn5JS0001', is_latest=True, key='my_analysis/dataset_subsetted.parquet', suffix='.parquet', kind='dataset', otype='DataFrame', size=3839, hash='s5a7sMZMWEXie9Oy-UDK5g', space_id=1, storage_id=1, run_id=4, created_by_id=1, created_at=2025-02-18 13:41:20 UTC); to track this artifact as an input, use: ln.Artifact.get()
→ found artifact with same hash: Artifact(uid='Xu8DxC35abee9ERp0000', is_latest=True, description='log streams of run Qvbucg6hc7fSfyQp0aK2', suffix='.txt', size=0, hash='1B2M2Y8AsgTpgAmY7PhCfg', space_id=1, storage_id=1, created_by_id=1, created_at=2025-02-18 13:41:16 UTC); to track this artifact as an input, use: ln.Artifact.get()
! updated description from log streams of run Qvbucg6hc7fSfyQp0aK2 to log streams of run uiMoQAnZPuk3OzmjA8la
→ finished Run('uiMoQAnZ') after 1s at 2025-02-18 13:41:26 UTC
ln.view()
Show code cell output
Artifact
uid | key | description | suffix | kind | otype | size | hash | n_files | n_observations | _hash_type | _key_is_virtual | _overwrite_versions | space_id | storage_id | schema_id | version | is_latest | run_id | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
id | |||||||||||||||||||||||
5 | GGCIFFTYUfmJn5JS0001 | my_analysis/dataset_subsetted.parquet | None | .parquet | dataset | DataFrame | 3839 | s5a7sMZMWEXie9Oy-UDK5g | None | None | md5 | True | False | 1 | 1 | None | None | True | 6 | 2025-02-18 13:41:20.424000+00:00 | 1 | None | 1 |
4 | GGCIFFTYUfmJn5JS0000 | my_analysis/dataset_subsetted.parquet | None | .parquet | dataset | DataFrame | 3233 | 7jKFlRPwu3gPRxVnkb6qaw | None | None | md5 | True | False | 1 | 1 | None | None | False | 3 | 2025-02-18 13:41:19.901000+00:00 | 1 | None | 1 |
3 | PhKAkwD9McPMysfR0000 | my_analysis/dataset.parquet | None | .parquet | dataset | DataFrame | 6586 | yMhflyJSY9zEHe9ew5-JqA | None | None | md5 | True | False | 1 | 1 | None | None | True | 5 | 2025-02-18 13:41:19.827000+00:00 | 1 | None | 1 |
Param
name | dtype | is_type | _expect_many | space_id | type_id | run_id | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|---|---|---|
id | |||||||||||
8 | run_workflow_subset | bool | None | False | 1 | None | NaN | 2025-02-18 13:41:24.650000+00:00 | 1 | None | 1 |
7 | output_artifact_key | str | None | False | 1 | None | 2.0 | 2025-02-18 13:41:19.763000+00:00 | 1 | None | 1 |
6 | input_artifact_key | str | None | False | 1 | None | 2.0 | 2025-02-18 13:41:19.756000+00:00 | 1 | None | 1 |
5 | subset_cols | int | None | False | 1 | None | 2.0 | 2025-02-18 13:41:19.749000+00:00 | 1 | None | 1 |
4 | subset_rows | int | None | False | 1 | None | 2.0 | 2025-02-18 13:41:19.742000+00:00 | 1 | None | 1 |
3 | preprocess_params | dict | None | False | 1 | None | NaN | 2025-02-18 13:41:10.129000+00:00 | 1 | None | 1 |
2 | learning_rate | float | None | False | 1 | None | NaN | 2025-02-18 13:41:10.123000+00:00 | 1 | None | 1 |
ParamValue
value | hash | space_id | param_id | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|
id | ||||||||
1 | ./mydataset | None | 1 | 1 | 2025-02-18 13:41:14.048000+00:00 | 1 | None | 1 |
2 | 0.01 | None | 1 | 2 | 2025-02-18 13:41:14.048000+00:00 | 1 | None | 1 |
3 | {'downsample': True, 'normalization': 'the_goo... | None | 1 | 3 | 2025-02-18 13:41:14.048000+00:00 | 1 | None | 1 |
4 | my_analysis/dataset.parquet | None | 1 | 6 | 2025-02-18 13:41:19.872000+00:00 | 1 | None | 1 |
5 | my_analysis/dataset_subsetted.parquet | None | 1 | 7 | 2025-02-18 13:41:19.872000+00:00 | 1 | None | 1 |
6 | 2 | None | 1 | 4 | 2025-02-18 13:41:19.872000+00:00 | 1 | None | 1 |
7 | 2 | None | 1 | 5 | 2025-02-18 13:41:19.872000+00:00 | 1 | None | 1 |
Run
uid | name | started_at | finished_at | reference | reference_type | _is_consecutive | _status_code | space_id | transform_id | report_id | _logfile_id | environment_id | initiated_by_run_id | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
id | ||||||||||||||||||
1 | Qvbucg6hc7fSfyQp0aK2 | None | 2025-02-18 13:41:14.027840+00:00 | 2025-02-18 13:41:16.065501+00:00 | None | None | True | 0 | 1 | 1 | 2.0 | None | 1.0 | NaN | 2025-02-18 13:41:14.028000+00:00 | 1 | None | 1 |
2 | lBVxeHILVLR7iB0vwCDL | None | 2025-02-18 13:41:18.252149+00:00 | NaT | None | None | None | 0 | 1 | 2 | NaN | None | NaN | NaN | 2025-02-18 13:41:18.253000+00:00 | 1 | None | 1 |
3 | K0mfvaqZti09j1AaJq44 | None | 2025-02-18 13:41:19.852146+00:00 | 2025-02-18 13:41:19.904506+00:00 | None | None | None | 0 | 1 | 3 | NaN | None | NaN | 2.0 | 2025-02-18 13:41:19.852000+00:00 | 1 | None | 1 |
4 | ayroJ8z2cuoa8xEZsbgM | None | 2025-02-18 13:41:20.364973+00:00 | 2025-02-18 13:41:20.429714+00:00 | None | None | None | 0 | 1 | 3 | NaN | None | NaN | 2.0 | 2025-02-18 13:41:20.365000+00:00 | 1 | None | 1 |
5 | uiMoQAnZPuk3OzmjA8la | None | 2025-02-18 13:41:24.667211+00:00 | 2025-02-18 13:41:26.278308+00:00 | None | None | True | 0 | 1 | 4 | 2.0 | None | 1.0 | NaN | 2025-02-18 13:41:24.669000+00:00 | 1 | None | 1 |
6 | JMANsjf9YAnWI2np8J0s | None | 2025-02-18 13:41:26.221976+00:00 | 2025-02-18 13:41:26.272929+00:00 | None | None | None | 0 | 1 | 5 | NaN | None | NaN | 5.0 | 2025-02-18 13:41:26.222000+00:00 | 1 | None | 1 |
Storage
uid | root | description | type | region | instance_uid | space_id | run_id | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|---|---|---|---|
id | ||||||||||||
1 | CPhhQrlufozK | /home/runner/work/lamindb/lamindb/docs/test-track | None | local | None | 73KPGC58ahU9 | 1 | None | 2025-02-18 13:41:04.256000+00:00 | 1 | None | 1 |
Transform
uid | key | description | type | source_code | hash | reference | reference_type | space_id | _template_id | version | is_latest | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
id | ||||||||||||||||
5 | iSQXxR4c0BOE0000 | run-workflow.py/subset_dataframe.py | None | function | @ln.tracked()\ndef subset_dataframe(\n arti... | Dqbr_hMfHs17EhbPXP_PyQ | None | None | 1 | None | None | True | 2025-02-18 13:41:26.218000+00:00 | 1 | None | 1 |
4 | US4rlnbl6eKX0000 | run-workflow.py | run-workflow.py | script | import argparse\nimport lamindb as ln\n\nln.Pa... | yqr8j5hTUulVRzv4J-o9SQ | None | None | 1 | None | None | True | 2025-02-18 13:41:24.663000+00:00 | 1 | None | 1 |
3 | r0W2E5c3booT0000 | track.ipynb/subset_dataframe.py | None | function | @ln.tracked()\ndef subset_dataframe(\n inpu... | F_wwrfFs6zmzMGVilG2Prg | None | None | 1 | None | None | True | 2025-02-18 13:41:19.848000+00:00 | 1 | None | 1 |
2 | Hgy4VKDv3anM0000 | track.ipynb | Track notebooks, scripts & functions | notebook | None | None | None | None | 1 | None | None | True | 2025-02-18 13:41:18.245000+00:00 | 1 | None | 1 |
1 | j7Ecw9WoDmgs0000 | run-track-with-params.py | run-track-with-params.py | script | import argparse\nimport lamindb as ln\n\nif __... | nRUs3ZjuVTbKtBmSXpVQ5A | None | None | 1 | None | None | True | 2025-02-18 13:41:14.024000+00:00 | 1 | None | 1 |
Show code cell content
# clean up test instance
!rm -r ./test-track
!lamin delete --force test-track
• deleting instance testuser1/test-track