Generate single-cell images¶
Here, we are going to process the previously ingested microscopy images with the scPortrait pipeline to generate single-cell images that we can use to asses autophagosome formation at a single-cell level.
import lamindb as ln
from collections.abc import Iterable
from pathlib import Path
from scportrait.pipeline.extraction import HDF5CellExtraction
from scportrait.pipeline.project import Project
from scportrait.pipeline.segmentation.workflows import CytosolSegmentationCellpose
ln.track()
Show code cell output
→ connected lamindb: testuser1/test-sc-imaging
/opt/hostedtoolcache/Python/3.12.9/x64/lib/python3.12/site-packages/mahotas/morph.py:315: SyntaxWarning: invalid escape sequence '\s'
'''
/opt/hostedtoolcache/Python/3.12.9/x64/lib/python3.12/site-packages/mahotas/features/texture.py:33: SyntaxWarning: invalid escape sequence '\|'
'''
/opt/hostedtoolcache/Python/3.12.9/x64/lib/python3.12/site-packages/mahotas/features/texture.py:158: SyntaxWarning: invalid escape sequence '\|'
'''
→ created Transform('7TK6aTKb7XmZ0000'), started new Run('5F6RMbrE...') at 2025-03-31 12:45:59 UTC
→ notebook imports: lamindb==1.3.1 scportrait==1.3.3
First, we query for the raw and annotated microscopy images.
input_images = (
ln.Artifact.filter(ulabels__name="autophagy imaging")
.filter(description__icontains="raw image")
.filter(suffix=".tif")
)
The experiment contains different genotypes (WT
and EI24KO
) that were treated differently (unstimulated
vs 14h Torin-1
).
For each condition, multiple clonal cell lines were imaged across multiple fields of view in all imaging channels.
We need to get single-cell images from each FOV indivdually and tag them with all of the appropriate metadata to identify genotype, treatment condition, clonal cell line and imaging experiment.
select_artifacts_df = (
ln.Artifact.filter(ulabels__name="autophagy imaging")
.filter(description__icontains="raw image")
.df(features=True)
)
display(select_artifacts_df.head())
conditions = list(set.union(*select_artifacts_df["stimulation"].values))
cell_line_clones = list(set.union(*select_artifacts_df["cell_line_clone"].values))
FOVs = list(set.union(*select_artifacts_df["FOV"].values))
Show code cell output
uid | key | description | study | imaged structure | microscope | magnification | FOV | channel | cell_line_clone | stimulation | genotype | resolution | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
id | |||||||||||||
2 | Md4OouMExlWS2YfZ0000 | input_data_imaging_usecase/images/Timepoint001... | raw image of U2OS cells stained for autophagy ... | {autophagy imaging} | {LckLip-mNeon} | {Opera Phenix} | {20X} | {FOV1} | {Alexa488} | {U2OS lcklip-mNeon mCherryLC3B clone 1} | {14h Torin-1} | {WT} | {0.597976081} |
3 | KKbVRkOjQ1jdA2fx0000 | input_data_imaging_usecase/images/Timepoint001... | raw image of U2OS cells stained for autophagy ... | {autophagy imaging} | {LckLip-mNeon} | {Opera Phenix} | {20X} | {FOV2} | {Alexa488} | {U2OS lcklip-mNeon mCherryLC3B clone 1} | {14h Torin-1} | {WT} | {0.597976081} |
4 | CiQYTBNZrj0CPejK0000 | input_data_imaging_usecase/images/Timepoint001... | raw image of U2OS cells stained for autophagy ... | {autophagy imaging} | {DNA} | {Opera Phenix} | {20X} | {FOV1} | {DAPI} | {U2OS lcklip-mNeon mCherryLC3B clone 1} | {14h Torin-1} | {WT} | {0.597976081} |
5 | W6tzE7JNiM80Ruho0000 | input_data_imaging_usecase/images/Timepoint001... | raw image of U2OS cells stained for autophagy ... | {autophagy imaging} | {DNA} | {Opera Phenix} | {20X} | {FOV2} | {DAPI} | {U2OS lcklip-mNeon mCherryLC3B clone 1} | {14h Torin-1} | {WT} | NaN |
6 | YGiNq6DPfIEjtt9j0000 | input_data_imaging_usecase/images/Timepoint001... | raw image of U2OS cells stained for autophagy ... | {autophagy imaging} | {mCherry-LC3B} | {Opera Phenix} | {20X} | {FOV1} | {mCherry} | {U2OS lcklip-mNeon mCherryLC3B clone 1} | {14h Torin-1} | {WT} | NaN |
Alternatively, query for the ULabel
directly:
conditions = ln.ULabel.filter(
links_artifact__feature__name="stimulation", artifacts__in=input_images
).distinct()
cell_line_clones = ln.ULabel.filter(
links_artifact__feature__name="cell_line_clone", artifacts__in=input_images
).distinct()
FOVs = ln.ULabel.filter(
links_artifact__feature__name="FOV", artifacts__in=input_images
).distinct()
By iterating through conditions, celllines and FOVs, we should only have the 3 images showing a single FOV to enable processing using ScPortrait.
# load config file for processing all datasets
config_file_af = ln.Artifact.using("scportrait/examples").get(
key="input_data_imaging_usecase/config.yml"
)
config_file_af.description = (
"config for scportrait for processing of cells stained for autophagy markers"
)
config_file_af.save()
# annotate the config file with the metadata relevant to the study
config_file_af.features.add_values(
{"study": "autophagy imaging", "artifact type": "scportrait config"}
)
Show code cell output
→ mapped records: ULabel(uid='PKiCEP1h')
→ transferred records: Artifact(uid='voi8szTkmKPiahUA0000'), ULabel(uid='hbGRGfA1'), Feature(uid='Kb0eQtlXapg2')
Let’s take a look at the processing of one example FOV.
# get input images for one example FOV
condition = conditions[0]
cellline = cell_line_clones[0]
FOV = FOVs[0]
images = (
input_images.filter(ulabels=condition)
.filter(ulabels=cellline)
.filter(ulabels=FOV)
.distinct()
)
# Perform quick sanity check that we only have images which share all of their attributed except channel and imaged structure
_features = []
values_to_ignore = ["channel", "imaged structure"]
for af in images:
features = af.features.get_values()
features = {
key: features[key] for key in features.keys() if key not in values_to_ignore
}
_features.append(features)
assert all(_features[0] == f for f in _features)
shared_features = _features[0]
# bring image paths into the correct order for processing
input_image_paths = [
images.filter(ulabels__name=channel_name).one().cache()
for channel_name in ["DAPI", "Alexa488", "mCherry"]
]
# define and create an output location for the processed data
output_directory = "processed_data"
Path(output_directory).mkdir(parents=True, exist_ok=True)
# initialize our scportrait project with a unique ID
unique_project_id = f"{shared_features['cell_line_clone']}/{shared_features['stimulation']}/{shared_features['FOV']}".replace(
" ", "_"
)
# create the project location
project_location = f"{output_directory}/{unique_project_id}/scportrait_project"
Path(project_location).mkdir(parents=True, exist_ok=True)
# initialize the project
project = Project(
project_location=project_location,
config_path=config_file_af.cache(),
segmentation_f=CytosolSegmentationCellpose,
extraction_f=HDF5CellExtraction,
overwrite=True,
)
# load our input images
project.load_input_from_tif_files(
input_image_paths, overwrite=True, channel_names=["DAPI", "Alexa488", "mCherry"]
)
# process the project
project.segment()
project.extract()
Show code cell output
Updating project config file.
INFO The Zarr backing store has been changed from None the new file path:
processed_data/U2OS_lcklip-mNeon_mCherryLC3B_clone_1/14h_Torin-1/FOV1/scportrait_project/scportrait.sdata
0%| | 0.00/25.3M [00:00<?, ?B/s]
0%| | 80.0k/25.3M [00:00<00:32, 804kB/s]
1%|▏ | 384k/25.3M [00:00<00:12, 2.10MB/s]
6%|▌ | 1.58M/25.3M [00:00<00:03, 6.71MB/s]
25%|██▌ | 6.39M/25.3M [00:00<00:00, 23.3MB/s]
52%|█████▏ | 13.1M/25.3M [00:00<00:00, 39.5MB/s]
69%|██████▉ | 17.6M/25.3M [00:00<00:00, 41.9MB/s]
86%|████████▌ | 21.8M/25.3M [00:00<00:00, 42.6MB/s]
100%|██████████| 25.3M/25.3M [00:00<00:00, 29.6MB/s]
0%| | 0.00/3.54k [00:00<?, ?B/s]
100%|██████████| 3.54k/3.54k [00:00<00:00, 8.25MB/s]
0%| | 0.00/25.3M [00:00<?, ?B/s]
0%| | 80.0k/25.3M [00:00<00:33, 794kB/s]
2%|▏ | 416k/25.3M [00:00<00:11, 2.27MB/s]
7%|▋ | 1.69M/25.3M [00:00<00:03, 7.13MB/s]
24%|██▍ | 6.19M/25.3M [00:00<00:00, 21.7MB/s]
54%|█████▎ | 13.6M/25.3M [00:00<00:00, 40.5MB/s]
81%|████████ | 20.5M/25.3M [00:00<00:00, 47.2MB/s]
100%|██████████| 25.3M/25.3M [00:00<00:00, 37.2MB/s]
0%| | 0.00/3.54k [00:00<?, ?B/s]
100%|██████████| 3.54k/3.54k [00:00<00:00, 17.0MB/s]
First, lets look at the input images we processed.
project.plot_input_image()
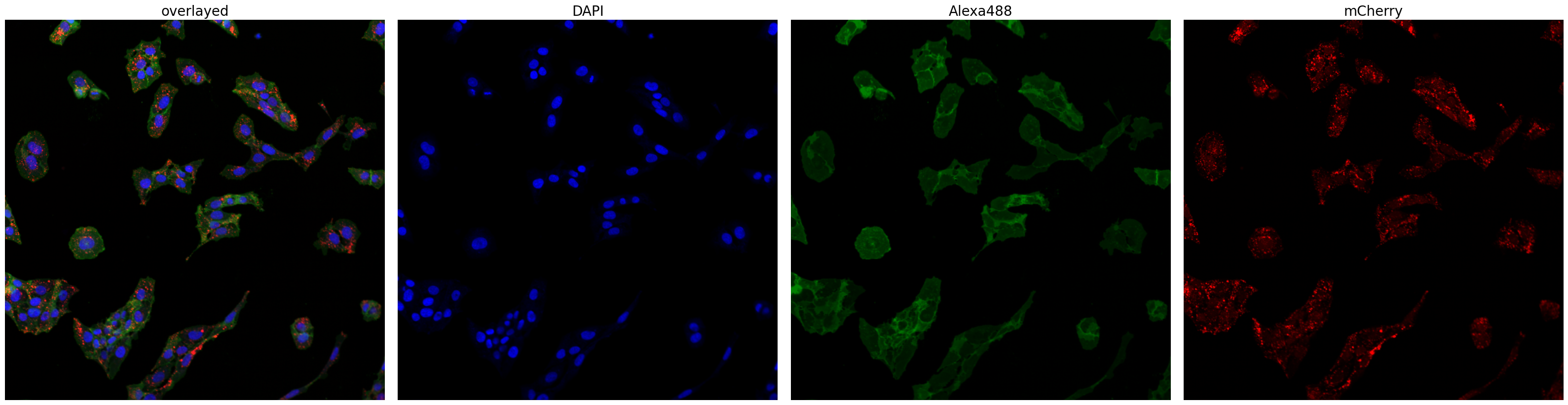
Now we can look at the results generated by scPortrait. First the segmentation masks.
project.plot_segmentation_masks()
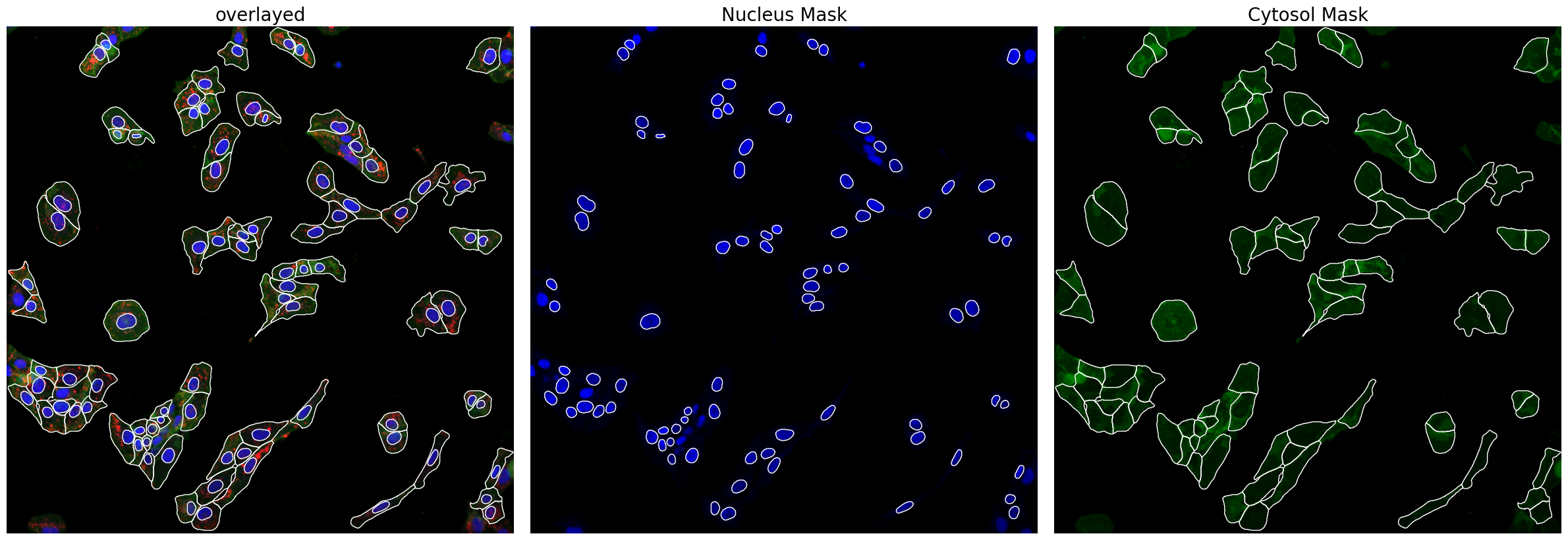
And then extraction results consisting of individual single-cell images over all of the channels.
project.plot_single_cell_images()
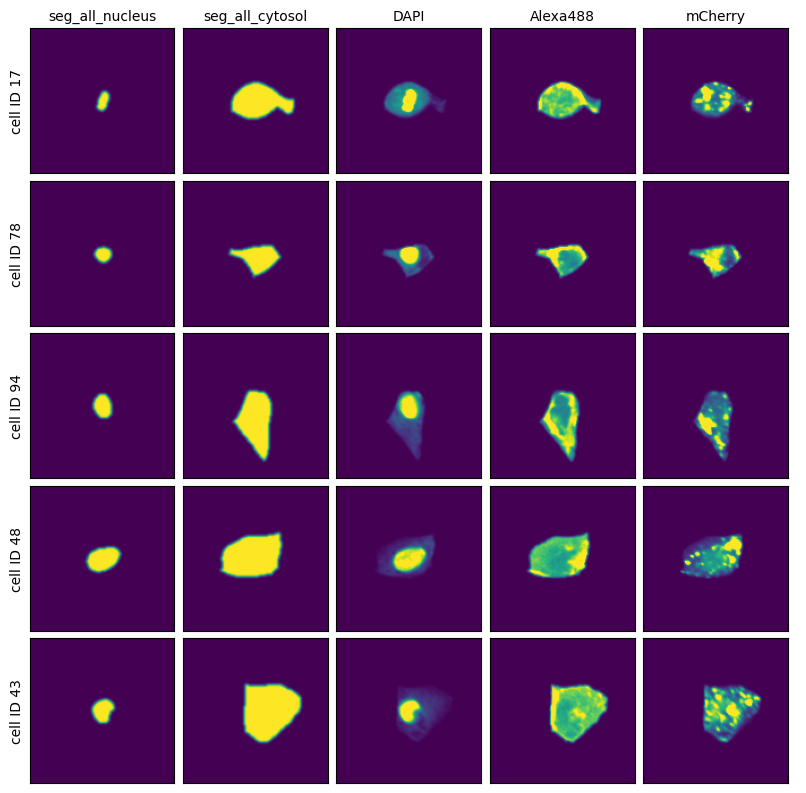
Now we also want to save these results to our instance.
ln.Artifact.from_spatialdata(
sdata=project.filehandler.get_sdata(),
description="scportrait spatialdata object containing results of cells stained for autophagy markers",
key=f"processed_data_imaging_use_case/{unique_project_id}/spatialdata.zarr",
).save()
Show code cell output
INFO The SpatialData object is not self-contained (i.e. it contains some elements that are Dask-backed from
locations outside /home/runner/.cache/lamindb/JbwN821h6PjaTKYH0000.zarr). Please see the documentation of
`is_self_contained()` to understand the implications of working with SpatialData objects that are not
self-contained.
INFO The Zarr backing store has been changed from
processed_data/U2OS_lcklip-mNeon_mCherryLC3B_clone_1/14h_Torin-1/FOV1/scportrait_project/scportrait.sdata
the new file path: /home/runner/.cache/lamindb/JbwN821h6PjaTKYH0000.zarr
Artifact(uid='JbwN821h6PjaTKYH0000', is_latest=True, key='processed_data_imaging_use_case/U2OS_lcklip-mNeon_mCherryLC3B_clone_1/14h_Torin-1/FOV1/spatialdata.zarr', description='scportrait spatialdata object containing results of cells stained for autophagy markers', suffix='.zarr', kind='dataset', otype='SpatialData', size=6973559, hash='bQyHXMSBxjyWuayNkwVI2g', n_files=69, space_id=1, storage_id=1, run_id=3, created_by_id=1, created_at=2025-03-31 12:47:28 UTC)
# define var schema
var_schema = ln.Schema(
name="single-cell image dataset schema var",
description="column schema for data measured in obsm[single_cell_images]",
itype=ln.Feature,
dtype=float,
).save()
# define obs schema
obs_schema = ln.Schema(
name="single-cell image dataset schema obs",
features=[
ln.Feature(name="scportrait_cell_id", dtype="int", coerce_dtype=True).save(),
],
).save()
# define uns schema
uns_schema = ln.Schema(
name="single-cell image dataset schema uns",
itype=ln.Feature,
dtype=dict,
).save()
# define composite schema
h5sc_schema = ln.Schema(
name="single-cell image dataset",
otype="AnnData",
components={"var": var_schema, "obs": obs_schema, "uns": uns_schema},
).save()
# curate an AnnData
curator = ln.curators.AnnDataCurator(project.h5sc, h5sc_schema)
curator.validate()
artifact = curator.save_artifact(
key=f"processed_data_imaging_use_case/{unique_project_id}/single_cell_data.h5ad"
)
# add shared annotation
annotation = shared_features.copy()
annotation["imaged structure"] = [
ln.ULabel.using("scportrait/examples").get(name=structure_name)
for structure_name in ["LckLip-mNeon", "DNA", "mCherry-LC3B"]
]
artifact.features.add_values(annotation)
artifact.labels.add(ln.ULabel(name="scportrait single-cell images").save())
Show code cell output
! record with similar name exists! did you mean to load it?
uid | name | description | n | dtype | itype | is_type | otype | hash | minimal_set | ordered_set | maximal_set | _curation | slot | space_id | type_id | validated_by_id | composite_id | run_id | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
id | |||||||||||||||||||||||
2 | r5TbSfdV6kqMzPRnGHNC | single-cell image dataset schema var | column schema for data measured in obsm[single... | -1 | float | Feature | False | None | 2LXd3Ex5pNOrDsqEiHkuVw | True | False | False | None | None | 1 | None | None | None | 3 | 2025-03-31 12:47:28.431000+00:00 | 1 | None | 1 |
! records with similar names exist! did you mean to load one of them?
uid | name | description | n | dtype | itype | is_type | otype | hash | minimal_set | ordered_set | maximal_set | _curation | slot | space_id | type_id | validated_by_id | composite_id | run_id | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
id | |||||||||||||||||||||||
2 | r5TbSfdV6kqMzPRnGHNC | single-cell image dataset schema var | column schema for data measured in obsm[single... | -1 | float | Feature | False | None | 2LXd3Ex5pNOrDsqEiHkuVw | True | False | False | None | None | 1 | None | None | None | 3 | 2025-03-31 12:47:28.431000+00:00 | 1 | None | 1 |
3 | 3Ajy5VY7H6PVoAhsFLfn | single-cell image dataset schema obs | None | 1 | None | Feature | False | None | 0Wb4Qaes_RIx5s4g5SWeDA | True | False | False | None | None | 1 | None | None | None | 3 | 2025-03-31 12:47:28.460000+00:00 | 1 | None | 1 |
! records with similar names exist! did you mean to load one of them?
uid | name | description | n | dtype | itype | is_type | otype | hash | minimal_set | ordered_set | maximal_set | _curation | slot | space_id | type_id | validated_by_id | composite_id | run_id | created_at | created_by_id | _aux | _branch_code | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
id | |||||||||||||||||||||||
2 | r5TbSfdV6kqMzPRnGHNC | single-cell image dataset schema var | column schema for data measured in obsm[single... | -1 | float | Feature | False | None | 2LXd3Ex5pNOrDsqEiHkuVw | True | False | False | None | None | 1 | None | None | None | 3 | 2025-03-31 12:47:28.431000+00:00 | 1 | None | 1 |
3 | 3Ajy5VY7H6PVoAhsFLfn | single-cell image dataset schema obs | None | 1 | None | Feature | False | None | 0Wb4Qaes_RIx5s4g5SWeDA | True | False | False | None | None | 1 | None | None | None | 3 | 2025-03-31 12:47:28.460000+00:00 | 1 | None | 1 |
4 | dfPbB8VufVTnU3vmr2LR | single-cell image dataset schema uns | None | -1 | dict | Feature | False | None | eM1K4iIPtsi5Lc7ONRL_aQ | True | False | False | None | None | 1 | None | None | None | 3 | 2025-03-31 12:47:28.483000+00:00 | 1 | None | 1 |
• path content will be copied to default storage upon `save()` with key 'processed_data_imaging_use_case/U2OS_lcklip-mNeon_mCherryLC3B_clone_1/14h_Torin-1/FOV1/single_cell_data.h5ad'
✓ storing artifact 'AUxDIEZ5VOaB6RtJ0000' at '/home/runner/work/lamin-usecases/lamin-usecases/docs/test-sc-imaging/.lamindb/AUxDIEZ5VOaB6RtJ0000.h5ad'
! 5 unique terms (100.00%) are not validated for name: '0', '1', '2', '3', '4'
✓ 1 unique term (100.00%) is validated for name
→ returning existing schema with same hash: Schema(uid='3Ajy5VY7H6PVoAhsFLfn', name='single-cell image dataset schema obs', n=1, itype='Feature', is_type=False, hash='0Wb4Qaes_RIx5s4g5SWeDA', minimal_set=True, ordered_set=False, maximal_set=False, space_id=1, created_by_id=1, run_id=3, created_at=2025-03-31 12:47:28 UTC)
! updated otype from None to DataFrame
! did not create Feature record for 1 non-validated name: 'single_cell_images'
To make our lives easier so that we can process all of the files in our dataset we are going to write a custom image processing function.
We decorate this function with :func:~lamindb.tracked
to track data lineage of the input and output Artifacts. To improve processing time we will only recompute datasets that have not been previously processed and uploaded to our instance.
@ln.tracked()
def _process_images(
config_file_af: ln.Artifact,
input_artifacts: Iterable[ln.Artifact],
h5sc_schema: ln.Schema,
output_directory: str,
) -> None:
# Perform quick sanity check that we only have images which share all of their attributes except channel and imaged structure
_features = []
values_to_ignore = ["channel", "imaged structure"]
for af in input_artifacts:
features = af.features.get_values()
features = {
key: features[key] for key in features.keys() if key not in values_to_ignore
}
_features.append(features)
assert all(_features[0] == f for f in _features)
shared_features = _features[0]
# create a unique identifier for the project based on the annotated features
unique_project_id = f"{shared_features['cell_line_clone']}/{shared_features['stimulation']}/{shared_features['FOV']}".replace(
" ", "_"
)
# check if processed results already exist and if so skip processing
try:
# check for single-cell images
(
ln.Artifact.using("scportrait/examples").get(
key=f"processed_data_imaging_use_case/{unique_project_id}/single_cell_data.h5ad"
)
)
# check for SpatialData object
(
ln.Artifact.using("scportrait/examples").get(
key=f"processed_data_imaging_use_case/{unique_project_id}/spatialdata.zarr"
)
)
print(
"Dataset already processed and results uploaded to instance. Skipping processing."
)
except ln.Artifact.DoesNotExist:
input_image_paths = [
input_artifacts.filter(ulabels__name=channel_name).one().cache()
for channel_name in ["DAPI", "Alexa488", "mCherry"]
]
# create the project location
project_location = f"{output_directory}/{unique_project_id}/scportrait_project"
Path(project_location).mkdir(parents=True, exist_ok=True)
project = Project(
project_location=project_location,
config_path=config_file_af.cache(),
segmentation_f=CytosolSegmentationCellpose,
extraction_f=HDF5CellExtraction,
overwrite=True,
)
# process the project
project.load_input_from_tif_files(
input_image_paths,
overwrite=True,
channel_names=["DAPI", "Alexa488", "mCherry"],
)
project.segment()
project.extract()
# ingest results to instance
# single-cell images
curator = ln.curators.AnnDataCurator(project.h5sc, h5sc_schema)
artifact = curator.save_artifact(
key=f"processed_data_imaging_use_case/{unique_project_id}/single_cell_data.h5ad"
)
annotation = shared_features.copy()
annotation["imaged structure"] = [
ln.ULabel.using("scportrait/examples").get(name=structure_name)
for structure_name in ["LckLip-mNeon", "DNA", "mCherry-LC3B"]
]
artifact.features.add_values(annotation)
artifact.labels.add(ln.ULabel.get(name="scportrait single-cell images"))
# SpatialData object
ln.Artifact.from_spatialdata(
sdata=project.filehandler.get_sdata(),
description="scportrait spatialdata object containing results of cells stained for autophagy markers",
key=f"processed_data_imaging_use_case/{unique_project_id}/spatialdata.zarr",
).save()
return None
ln.Param(name="output_directory", dtype="str").save()
Show code cell output
Param(name='output_directory', dtype='str', space_id=1, created_by_id=1, run_id=3, created_at=2025-03-31 12:47:31 UTC)
Now we are ready to process all of our input images and upload the generated single-cell image datasets back to our instance.
for condition in conditions:
for cellline in cell_line_clones:
for FOV in FOVs:
images = (
input_images.filter(ulabels=condition)
.filter(ulabels=cellline)
.filter(ulabels=FOV)
.distinct()
)
if images:
_process_images(
config_file_af,
input_artifacts=images,
h5sc_schema=h5sc_schema,
output_directory=output_directory,
)
Show code cell output
! cannot infer feature type of: <QuerySet [Artifact(uid='Md4OouMExlWS2YfZ0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_Alexa488_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='0aoXxT857VvKAGo9UQo-8g', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='CiQYTBNZrj0CPejK0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_DAPI_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='YRjhTt2cBLq3BukWLUKC_w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='YGiNq6DPfIEjtt9j0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_mCherry_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='zptjd_tR7P2Nw6mjUWbEjw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Md4OouMExlWS2YfZ0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_Alexa488_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='0aoXxT857VvKAGo9UQo-8g', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='CiQYTBNZrj0CPejK0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_DAPI_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='YRjhTt2cBLq3BukWLUKC_w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='YGiNq6DPfIEjtt9j0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_mCherry_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='zptjd_tR7P2Nw6mjUWbEjw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='KKbVRkOjQ1jdA2fx0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_Alexa488_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='Mm-DsaVdkMbTreUW_ipQBQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='W6tzE7JNiM80Ruho0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_DAPI_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9_LVWK08Z_D4c1mvIJlTOA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='uuh41FAHEz0ASL2N0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_mCherry_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='PSnZ2s1_uJa9G4_cc2HpNw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='KKbVRkOjQ1jdA2fx0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_Alexa488_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='Mm-DsaVdkMbTreUW_ipQBQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='W6tzE7JNiM80Ruho0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_DAPI_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9_LVWK08Z_D4c1mvIJlTOA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='uuh41FAHEz0ASL2N0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_mCherry_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='PSnZ2s1_uJa9G4_cc2HpNw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Md4OouMExlWS2YfZ0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_Alexa488_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='0aoXxT857VvKAGo9UQo-8g', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='CiQYTBNZrj0CPejK0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_DAPI_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='YRjhTt2cBLq3BukWLUKC_w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='YGiNq6DPfIEjtt9j0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_mCherry_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='zptjd_tR7P2Nw6mjUWbEjw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Md4OouMExlWS2YfZ0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_Alexa488_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='0aoXxT857VvKAGo9UQo-8g', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='CiQYTBNZrj0CPejK0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_DAPI_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='YRjhTt2cBLq3BukWLUKC_w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='YGiNq6DPfIEjtt9j0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_mCherry_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='zptjd_tR7P2Nw6mjUWbEjw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='KKbVRkOjQ1jdA2fx0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_Alexa488_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='Mm-DsaVdkMbTreUW_ipQBQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='W6tzE7JNiM80Ruho0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_DAPI_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9_LVWK08Z_D4c1mvIJlTOA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='uuh41FAHEz0ASL2N0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_mCherry_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='PSnZ2s1_uJa9G4_cc2HpNw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='KKbVRkOjQ1jdA2fx0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_Alexa488_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='Mm-DsaVdkMbTreUW_ipQBQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='W6tzE7JNiM80Ruho0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_DAPI_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9_LVWK08Z_D4c1mvIJlTOA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='uuh41FAHEz0ASL2N0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_mCherry_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='PSnZ2s1_uJa9G4_cc2HpNw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='yiIMSAddDWgLgki70000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_Alexa488_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='KUhrzT6sDoICDg9cGCUkmg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='DEzw4QQAsjVZ010b0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_DAPI_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='N2xVJ8pQ9jWoywub8om_iQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='hbVyCGFARHU91Kax0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_mCherry_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='U2Hrdj8bUugok31k_uqEuw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='yiIMSAddDWgLgki70000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_Alexa488_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='KUhrzT6sDoICDg9cGCUkmg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='DEzw4QQAsjVZ010b0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_DAPI_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='N2xVJ8pQ9jWoywub8om_iQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='hbVyCGFARHU91Kax0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_mCherry_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='U2Hrdj8bUugok31k_uqEuw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='2ie2Kjzn1O7UYhuq0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_Alexa488_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='xkGVufN-7DDAZOeh4h7LpA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='fOQSb7JCK67aeN6a0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_DAPI_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='aNYMykHkxezUxs-07xgikw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='qHQpdWcFu7FzF6l50000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_mCherry_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='_eUmLccyLWPhCAZBQ8a3zA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='2ie2Kjzn1O7UYhuq0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_Alexa488_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='xkGVufN-7DDAZOeh4h7LpA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='fOQSb7JCK67aeN6a0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_DAPI_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='aNYMykHkxezUxs-07xgikw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='qHQpdWcFu7FzF6l50000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_mCherry_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='_eUmLccyLWPhCAZBQ8a3zA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='yiIMSAddDWgLgki70000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_Alexa488_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='KUhrzT6sDoICDg9cGCUkmg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='DEzw4QQAsjVZ010b0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_DAPI_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='N2xVJ8pQ9jWoywub8om_iQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='hbVyCGFARHU91Kax0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_mCherry_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='U2Hrdj8bUugok31k_uqEuw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='yiIMSAddDWgLgki70000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_Alexa488_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='KUhrzT6sDoICDg9cGCUkmg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='DEzw4QQAsjVZ010b0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_DAPI_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='N2xVJ8pQ9jWoywub8om_iQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='hbVyCGFARHU91Kax0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_mCherry_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='U2Hrdj8bUugok31k_uqEuw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='2ie2Kjzn1O7UYhuq0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_Alexa488_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='xkGVufN-7DDAZOeh4h7LpA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='fOQSb7JCK67aeN6a0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_DAPI_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='aNYMykHkxezUxs-07xgikw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='qHQpdWcFu7FzF6l50000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_mCherry_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='_eUmLccyLWPhCAZBQ8a3zA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='2ie2Kjzn1O7UYhuq0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_Alexa488_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='xkGVufN-7DDAZOeh4h7LpA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='fOQSb7JCK67aeN6a0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_DAPI_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='aNYMykHkxezUxs-07xgikw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='qHQpdWcFu7FzF6l50000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_mCherry_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='_eUmLccyLWPhCAZBQ8a3zA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='jVytS8AyAHmHkYR30000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_Alexa488_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='QFmofkisy-sibXAuKrpfRw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='cw4F6bUB9zuMthCY0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_DAPI_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='f4Vij96NOq7M96SXfyEYXQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='7TZGXvbA0JLL68hR0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_mCherry_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='qUKQaesz-vfPUwljqPlIjA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='jVytS8AyAHmHkYR30000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_Alexa488_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='QFmofkisy-sibXAuKrpfRw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='cw4F6bUB9zuMthCY0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_DAPI_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='f4Vij96NOq7M96SXfyEYXQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='7TZGXvbA0JLL68hR0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_mCherry_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='qUKQaesz-vfPUwljqPlIjA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='vCVbKkzz4CnJPPKF0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_Alexa488_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='WyabAqK4jeZtTtrQ9rvUeQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='5kMhlcDNek4RMeQF0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_DAPI_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='eXaBPwLJJ--9SyGD7JR0ew', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='9BmbViqMmlVhpfS00000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_mCherry_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='EeQ_zoM4j8waIP8wP-u0Hg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='vCVbKkzz4CnJPPKF0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_Alexa488_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='WyabAqK4jeZtTtrQ9rvUeQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='5kMhlcDNek4RMeQF0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_DAPI_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='eXaBPwLJJ--9SyGD7JR0ew', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='9BmbViqMmlVhpfS00000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_mCherry_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='EeQ_zoM4j8waIP8wP-u0Hg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='jVytS8AyAHmHkYR30000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_Alexa488_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='QFmofkisy-sibXAuKrpfRw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='cw4F6bUB9zuMthCY0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_DAPI_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='f4Vij96NOq7M96SXfyEYXQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='7TZGXvbA0JLL68hR0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_mCherry_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='qUKQaesz-vfPUwljqPlIjA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='jVytS8AyAHmHkYR30000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_Alexa488_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='QFmofkisy-sibXAuKrpfRw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='cw4F6bUB9zuMthCY0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_DAPI_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='f4Vij96NOq7M96SXfyEYXQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='7TZGXvbA0JLL68hR0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_mCherry_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='qUKQaesz-vfPUwljqPlIjA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='vCVbKkzz4CnJPPKF0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_Alexa488_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='WyabAqK4jeZtTtrQ9rvUeQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='5kMhlcDNek4RMeQF0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_DAPI_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='eXaBPwLJJ--9SyGD7JR0ew', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='9BmbViqMmlVhpfS00000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_mCherry_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='EeQ_zoM4j8waIP8wP-u0Hg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='vCVbKkzz4CnJPPKF0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_Alexa488_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='WyabAqK4jeZtTtrQ9rvUeQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='5kMhlcDNek4RMeQF0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_DAPI_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='eXaBPwLJJ--9SyGD7JR0ew', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='9BmbViqMmlVhpfS00000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_mCherry_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='EeQ_zoM4j8waIP8wP-u0Hg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='OS0wBE7bviIlW7qj0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_Alexa488_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='RwasAYhkUEeTVBFrwCbKuw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='ixOpuSTsyrPXdYuA0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_DAPI_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='diUYJPHgU_SvK0zTrrzUlw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='6uMjKAk1aYlAV7Cf0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_mCherry_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9K0N7dMe59K6zYUtjE74cA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='OS0wBE7bviIlW7qj0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_Alexa488_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='RwasAYhkUEeTVBFrwCbKuw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='ixOpuSTsyrPXdYuA0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_DAPI_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='diUYJPHgU_SvK0zTrrzUlw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='6uMjKAk1aYlAV7Cf0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_mCherry_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9K0N7dMe59K6zYUtjE74cA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='9ZVngbl0JUS0XdZ70000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_Alexa488_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='W1Q1RQCUj7j4e9R5Sta4wQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='IzP3IAwIhmM7OORD0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_DAPI_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='dIUw6okO0jOq6JcgsxDktA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='RRVS8qVx3VSw02Xu0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_mCherry_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9ZmlWOdgh9GFPd4BCYO7GQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='9ZVngbl0JUS0XdZ70000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_Alexa488_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='W1Q1RQCUj7j4e9R5Sta4wQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='IzP3IAwIhmM7OORD0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_DAPI_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='dIUw6okO0jOq6JcgsxDktA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='RRVS8qVx3VSw02Xu0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_mCherry_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9ZmlWOdgh9GFPd4BCYO7GQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='OS0wBE7bviIlW7qj0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_Alexa488_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='RwasAYhkUEeTVBFrwCbKuw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='ixOpuSTsyrPXdYuA0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_DAPI_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='diUYJPHgU_SvK0zTrrzUlw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='6uMjKAk1aYlAV7Cf0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_mCherry_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9K0N7dMe59K6zYUtjE74cA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='OS0wBE7bviIlW7qj0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_Alexa488_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='RwasAYhkUEeTVBFrwCbKuw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='ixOpuSTsyrPXdYuA0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_DAPI_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='diUYJPHgU_SvK0zTrrzUlw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='6uMjKAk1aYlAV7Cf0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_mCherry_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9K0N7dMe59K6zYUtjE74cA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='9ZVngbl0JUS0XdZ70000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_Alexa488_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='W1Q1RQCUj7j4e9R5Sta4wQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='IzP3IAwIhmM7OORD0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_DAPI_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='dIUw6okO0jOq6JcgsxDktA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='RRVS8qVx3VSw02Xu0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_mCherry_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9ZmlWOdgh9GFPd4BCYO7GQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='9ZVngbl0JUS0XdZ70000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_Alexa488_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='W1Q1RQCUj7j4e9R5Sta4wQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='IzP3IAwIhmM7OORD0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_DAPI_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='dIUw6okO0jOq6JcgsxDktA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='RRVS8qVx3VSw02Xu0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_mCherry_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9ZmlWOdgh9GFPd4BCYO7GQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Md4OouMExlWS2YfZ0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_Alexa488_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='0aoXxT857VvKAGo9UQo-8g', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='CiQYTBNZrj0CPejK0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_DAPI_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='YRjhTt2cBLq3BukWLUKC_w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='YGiNq6DPfIEjtt9j0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_mCherry_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='zptjd_tR7P2Nw6mjUWbEjw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Md4OouMExlWS2YfZ0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_Alexa488_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='0aoXxT857VvKAGo9UQo-8g', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='CiQYTBNZrj0CPejK0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_DAPI_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='YRjhTt2cBLq3BukWLUKC_w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='YGiNq6DPfIEjtt9j0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_mCherry_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='zptjd_tR7P2Nw6mjUWbEjw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='KKbVRkOjQ1jdA2fx0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_Alexa488_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='Mm-DsaVdkMbTreUW_ipQBQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='W6tzE7JNiM80Ruho0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_DAPI_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9_LVWK08Z_D4c1mvIJlTOA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='uuh41FAHEz0ASL2N0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_mCherry_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='PSnZ2s1_uJa9G4_cc2HpNw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='KKbVRkOjQ1jdA2fx0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_Alexa488_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='Mm-DsaVdkMbTreUW_ipQBQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='W6tzE7JNiM80Ruho0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_DAPI_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9_LVWK08Z_D4c1mvIJlTOA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='uuh41FAHEz0ASL2N0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_mCherry_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='PSnZ2s1_uJa9G4_cc2HpNw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Md4OouMExlWS2YfZ0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_Alexa488_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='0aoXxT857VvKAGo9UQo-8g', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='CiQYTBNZrj0CPejK0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_DAPI_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='YRjhTt2cBLq3BukWLUKC_w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='YGiNq6DPfIEjtt9j0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_mCherry_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='zptjd_tR7P2Nw6mjUWbEjw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Md4OouMExlWS2YfZ0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_Alexa488_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='0aoXxT857VvKAGo9UQo-8g', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='CiQYTBNZrj0CPejK0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_DAPI_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='YRjhTt2cBLq3BukWLUKC_w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='YGiNq6DPfIEjtt9j0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_mCherry_zstack001_r003_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='zptjd_tR7P2Nw6mjUWbEjw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='KKbVRkOjQ1jdA2fx0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_Alexa488_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='Mm-DsaVdkMbTreUW_ipQBQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='W6tzE7JNiM80Ruho0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_DAPI_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9_LVWK08Z_D4c1mvIJlTOA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='uuh41FAHEz0ASL2N0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_mCherry_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='PSnZ2s1_uJa9G4_cc2HpNw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='KKbVRkOjQ1jdA2fx0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_Alexa488_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='Mm-DsaVdkMbTreUW_ipQBQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='W6tzE7JNiM80Ruho0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_DAPI_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9_LVWK08Z_D4c1mvIJlTOA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='uuh41FAHEz0ASL2N0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well01_mCherry_zstack001_r004_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='PSnZ2s1_uJa9G4_cc2HpNw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='yiIMSAddDWgLgki70000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_Alexa488_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='KUhrzT6sDoICDg9cGCUkmg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='DEzw4QQAsjVZ010b0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_DAPI_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='N2xVJ8pQ9jWoywub8om_iQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='hbVyCGFARHU91Kax0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_mCherry_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='U2Hrdj8bUugok31k_uqEuw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='yiIMSAddDWgLgki70000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_Alexa488_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='KUhrzT6sDoICDg9cGCUkmg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='DEzw4QQAsjVZ010b0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_DAPI_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='N2xVJ8pQ9jWoywub8om_iQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='hbVyCGFARHU91Kax0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_mCherry_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='U2Hrdj8bUugok31k_uqEuw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='2ie2Kjzn1O7UYhuq0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_Alexa488_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='xkGVufN-7DDAZOeh4h7LpA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='fOQSb7JCK67aeN6a0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_DAPI_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='aNYMykHkxezUxs-07xgikw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='qHQpdWcFu7FzF6l50000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_mCherry_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='_eUmLccyLWPhCAZBQ8a3zA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='2ie2Kjzn1O7UYhuq0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_Alexa488_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='xkGVufN-7DDAZOeh4h7LpA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='fOQSb7JCK67aeN6a0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_DAPI_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='aNYMykHkxezUxs-07xgikw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='qHQpdWcFu7FzF6l50000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_mCherry_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='_eUmLccyLWPhCAZBQ8a3zA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='yiIMSAddDWgLgki70000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_Alexa488_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='KUhrzT6sDoICDg9cGCUkmg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='DEzw4QQAsjVZ010b0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_DAPI_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='N2xVJ8pQ9jWoywub8om_iQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='hbVyCGFARHU91Kax0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_mCherry_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='U2Hrdj8bUugok31k_uqEuw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='yiIMSAddDWgLgki70000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_Alexa488_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='KUhrzT6sDoICDg9cGCUkmg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='DEzw4QQAsjVZ010b0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_DAPI_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='N2xVJ8pQ9jWoywub8om_iQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='hbVyCGFARHU91Kax0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_mCherry_zstack001_r005_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='U2Hrdj8bUugok31k_uqEuw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='2ie2Kjzn1O7UYhuq0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_Alexa488_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='xkGVufN-7DDAZOeh4h7LpA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='fOQSb7JCK67aeN6a0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_DAPI_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='aNYMykHkxezUxs-07xgikw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='qHQpdWcFu7FzF6l50000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_mCherry_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='_eUmLccyLWPhCAZBQ8a3zA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='2ie2Kjzn1O7UYhuq0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_Alexa488_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='xkGVufN-7DDAZOeh4h7LpA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='fOQSb7JCK67aeN6a0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_DAPI_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='aNYMykHkxezUxs-07xgikw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='qHQpdWcFu7FzF6l50000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well01_mCherry_zstack001_r008_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='_eUmLccyLWPhCAZBQ8a3zA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='jVytS8AyAHmHkYR30000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_Alexa488_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='QFmofkisy-sibXAuKrpfRw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='cw4F6bUB9zuMthCY0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_DAPI_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='f4Vij96NOq7M96SXfyEYXQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='7TZGXvbA0JLL68hR0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_mCherry_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='qUKQaesz-vfPUwljqPlIjA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='jVytS8AyAHmHkYR30000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_Alexa488_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='QFmofkisy-sibXAuKrpfRw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='cw4F6bUB9zuMthCY0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_DAPI_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='f4Vij96NOq7M96SXfyEYXQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='7TZGXvbA0JLL68hR0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_mCherry_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='qUKQaesz-vfPUwljqPlIjA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='vCVbKkzz4CnJPPKF0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_Alexa488_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='WyabAqK4jeZtTtrQ9rvUeQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='5kMhlcDNek4RMeQF0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_DAPI_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='eXaBPwLJJ--9SyGD7JR0ew', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='9BmbViqMmlVhpfS00000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_mCherry_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='EeQ_zoM4j8waIP8wP-u0Hg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='vCVbKkzz4CnJPPKF0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_Alexa488_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='WyabAqK4jeZtTtrQ9rvUeQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='5kMhlcDNek4RMeQF0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_DAPI_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='eXaBPwLJJ--9SyGD7JR0ew', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='9BmbViqMmlVhpfS00000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_mCherry_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='EeQ_zoM4j8waIP8wP-u0Hg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='jVytS8AyAHmHkYR30000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_Alexa488_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='QFmofkisy-sibXAuKrpfRw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='cw4F6bUB9zuMthCY0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_DAPI_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='f4Vij96NOq7M96SXfyEYXQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='7TZGXvbA0JLL68hR0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_mCherry_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='qUKQaesz-vfPUwljqPlIjA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='jVytS8AyAHmHkYR30000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_Alexa488_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='QFmofkisy-sibXAuKrpfRw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='cw4F6bUB9zuMthCY0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_DAPI_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='f4Vij96NOq7M96SXfyEYXQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='7TZGXvbA0JLL68hR0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_mCherry_zstack001_r001_c007.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='qUKQaesz-vfPUwljqPlIjA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='vCVbKkzz4CnJPPKF0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_Alexa488_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='WyabAqK4jeZtTtrQ9rvUeQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='5kMhlcDNek4RMeQF0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_DAPI_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='eXaBPwLJJ--9SyGD7JR0ew', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='9BmbViqMmlVhpfS00000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_mCherry_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='EeQ_zoM4j8waIP8wP-u0Hg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='vCVbKkzz4CnJPPKF0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_Alexa488_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='WyabAqK4jeZtTtrQ9rvUeQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='5kMhlcDNek4RMeQF0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_DAPI_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='eXaBPwLJJ--9SyGD7JR0ew', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='9BmbViqMmlVhpfS00000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well01_mCherry_zstack001_r001_c008.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='EeQ_zoM4j8waIP8wP-u0Hg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='OS0wBE7bviIlW7qj0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_Alexa488_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='RwasAYhkUEeTVBFrwCbKuw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='ixOpuSTsyrPXdYuA0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_DAPI_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='diUYJPHgU_SvK0zTrrzUlw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='6uMjKAk1aYlAV7Cf0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_mCherry_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9K0N7dMe59K6zYUtjE74cA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='OS0wBE7bviIlW7qj0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_Alexa488_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='RwasAYhkUEeTVBFrwCbKuw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='ixOpuSTsyrPXdYuA0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_DAPI_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='diUYJPHgU_SvK0zTrrzUlw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='6uMjKAk1aYlAV7Cf0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_mCherry_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9K0N7dMe59K6zYUtjE74cA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='9ZVngbl0JUS0XdZ70000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_Alexa488_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='W1Q1RQCUj7j4e9R5Sta4wQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='IzP3IAwIhmM7OORD0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_DAPI_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='dIUw6okO0jOq6JcgsxDktA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='RRVS8qVx3VSw02Xu0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_mCherry_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9ZmlWOdgh9GFPd4BCYO7GQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='9ZVngbl0JUS0XdZ70000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_Alexa488_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='W1Q1RQCUj7j4e9R5Sta4wQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='IzP3IAwIhmM7OORD0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_DAPI_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='dIUw6okO0jOq6JcgsxDktA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='RRVS8qVx3VSw02Xu0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_mCherry_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9ZmlWOdgh9GFPd4BCYO7GQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='OS0wBE7bviIlW7qj0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_Alexa488_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='RwasAYhkUEeTVBFrwCbKuw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='ixOpuSTsyrPXdYuA0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_DAPI_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='diUYJPHgU_SvK0zTrrzUlw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='6uMjKAk1aYlAV7Cf0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_mCherry_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9K0N7dMe59K6zYUtjE74cA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='OS0wBE7bviIlW7qj0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_Alexa488_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='RwasAYhkUEeTVBFrwCbKuw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='ixOpuSTsyrPXdYuA0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_DAPI_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='diUYJPHgU_SvK0zTrrzUlw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='6uMjKAk1aYlAV7Cf0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_mCherry_zstack001_r001_c002.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9K0N7dMe59K6zYUtjE74cA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='9ZVngbl0JUS0XdZ70000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_Alexa488_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='W1Q1RQCUj7j4e9R5Sta4wQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='IzP3IAwIhmM7OORD0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_DAPI_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='dIUw6okO0jOq6JcgsxDktA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='RRVS8qVx3VSw02Xu0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_mCherry_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9ZmlWOdgh9GFPd4BCYO7GQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='9ZVngbl0JUS0XdZ70000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_Alexa488_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='W1Q1RQCUj7j4e9R5Sta4wQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='IzP3IAwIhmM7OORD0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_DAPI_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='dIUw6okO0jOq6JcgsxDktA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='RRVS8qVx3VSw02Xu0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well01_mCherry_zstack001_r001_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9ZmlWOdgh9GFPd4BCYO7GQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Gtwi9Pcyx8maQEWB0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_Alexa488_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='yJMlFFYyup0JmBu_FjTa3g', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='sHHpiiFYWsIXMZNV0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_DAPI_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='6pvuFREF3CQ8xr8Urak52Q', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='hNMkrIHce1XrLZHY0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_mCherry_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='K1jGiIE0dljR_qYP1btlyw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Gtwi9Pcyx8maQEWB0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_Alexa488_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='yJMlFFYyup0JmBu_FjTa3g', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='sHHpiiFYWsIXMZNV0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_DAPI_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='6pvuFREF3CQ8xr8Urak52Q', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='hNMkrIHce1XrLZHY0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_mCherry_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='K1jGiIE0dljR_qYP1btlyw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='nSZhAypqiNZ2Ylbe0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_Alexa488_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='uh537K7rMhMNKW-mti1vjw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='jzqxtoduIJ3hCbB40000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_DAPI_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='SOxM7EH80KPXK3hzBxXYLg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='M06liaIzh2OVEuJ40000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_mCherry_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='0tV4uQYxnP6G8JOrAwMyXw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='nSZhAypqiNZ2Ylbe0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_Alexa488_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='uh537K7rMhMNKW-mti1vjw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='jzqxtoduIJ3hCbB40000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_DAPI_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='SOxM7EH80KPXK3hzBxXYLg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='M06liaIzh2OVEuJ40000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_mCherry_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='0tV4uQYxnP6G8JOrAwMyXw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Gtwi9Pcyx8maQEWB0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_Alexa488_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='yJMlFFYyup0JmBu_FjTa3g', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='sHHpiiFYWsIXMZNV0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_DAPI_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='6pvuFREF3CQ8xr8Urak52Q', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='hNMkrIHce1XrLZHY0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_mCherry_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='K1jGiIE0dljR_qYP1btlyw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Gtwi9Pcyx8maQEWB0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_Alexa488_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='yJMlFFYyup0JmBu_FjTa3g', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='sHHpiiFYWsIXMZNV0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_DAPI_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='6pvuFREF3CQ8xr8Urak52Q', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='hNMkrIHce1XrLZHY0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_mCherry_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='K1jGiIE0dljR_qYP1btlyw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='nSZhAypqiNZ2Ylbe0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_Alexa488_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='uh537K7rMhMNKW-mti1vjw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='jzqxtoduIJ3hCbB40000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_DAPI_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='SOxM7EH80KPXK3hzBxXYLg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='M06liaIzh2OVEuJ40000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_mCherry_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='0tV4uQYxnP6G8JOrAwMyXw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='nSZhAypqiNZ2Ylbe0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_Alexa488_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='uh537K7rMhMNKW-mti1vjw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='jzqxtoduIJ3hCbB40000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_DAPI_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='SOxM7EH80KPXK3hzBxXYLg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='M06liaIzh2OVEuJ40000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_mCherry_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='0tV4uQYxnP6G8JOrAwMyXw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='mXWwV1x42Jz9RoSO0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_Alexa488_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='4xc4bpAMo0UaM_tc8SciIA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='gj0HHnoVpEqbaUJb0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_DAPI_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='fZ4W2ePHagfGUGCcve98gQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='1XnEyqVt6UGXCTmV0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_mCherry_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='if3WCKH91_CY0ptIVYuLmA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='mXWwV1x42Jz9RoSO0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_Alexa488_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='4xc4bpAMo0UaM_tc8SciIA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='gj0HHnoVpEqbaUJb0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_DAPI_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='fZ4W2ePHagfGUGCcve98gQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='1XnEyqVt6UGXCTmV0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_mCherry_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='if3WCKH91_CY0ptIVYuLmA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Ov8FnKzHMNY0XVJa0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_Alexa488_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='5cfc02GejBaoJ0h3OLWSdw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='Lwk8shsYe0V5bMgd0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_DAPI_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='CmAa139DH_lQ2Plcy4BxXw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='YuyVn060M4FxATPz0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_mCherry_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='gZHwk76xPz7qXiST4RuVMQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Ov8FnKzHMNY0XVJa0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_Alexa488_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='5cfc02GejBaoJ0h3OLWSdw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='Lwk8shsYe0V5bMgd0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_DAPI_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='CmAa139DH_lQ2Plcy4BxXw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='YuyVn060M4FxATPz0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_mCherry_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='gZHwk76xPz7qXiST4RuVMQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='mXWwV1x42Jz9RoSO0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_Alexa488_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='4xc4bpAMo0UaM_tc8SciIA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='gj0HHnoVpEqbaUJb0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_DAPI_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='fZ4W2ePHagfGUGCcve98gQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='1XnEyqVt6UGXCTmV0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_mCherry_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='if3WCKH91_CY0ptIVYuLmA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='mXWwV1x42Jz9RoSO0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_Alexa488_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='4xc4bpAMo0UaM_tc8SciIA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='gj0HHnoVpEqbaUJb0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_DAPI_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='fZ4W2ePHagfGUGCcve98gQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='1XnEyqVt6UGXCTmV0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_mCherry_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='if3WCKH91_CY0ptIVYuLmA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Ov8FnKzHMNY0XVJa0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_Alexa488_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='5cfc02GejBaoJ0h3OLWSdw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='Lwk8shsYe0V5bMgd0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_DAPI_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='CmAa139DH_lQ2Plcy4BxXw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='YuyVn060M4FxATPz0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_mCherry_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='gZHwk76xPz7qXiST4RuVMQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Ov8FnKzHMNY0XVJa0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_Alexa488_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='5cfc02GejBaoJ0h3OLWSdw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='Lwk8shsYe0V5bMgd0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_DAPI_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='CmAa139DH_lQ2Plcy4BxXw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='YuyVn060M4FxATPz0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_mCherry_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='gZHwk76xPz7qXiST4RuVMQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='ThuJnRAhqkp54kyU0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_Alexa488_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='vs3t8d40w259zgJRltHlKA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='PquYNyshQTDd24Vw0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_DAPI_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='1mSZ_MMg393bfTvMOl3YMQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='8SFUmW0RhBNySxBO0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_mCherry_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='fjO8ly0euUPHXoQd86weww', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='ThuJnRAhqkp54kyU0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_Alexa488_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='vs3t8d40w259zgJRltHlKA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='PquYNyshQTDd24Vw0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_DAPI_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='1mSZ_MMg393bfTvMOl3YMQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='8SFUmW0RhBNySxBO0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_mCherry_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='fjO8ly0euUPHXoQd86weww', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='h4EKWveW36LIzXez0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_Alexa488_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='ZPUWq_TI3KWNcVeVz3vOPg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='fdem35nw5ztUnEIM0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_DAPI_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='XuugTTV9S5TbJKVMLOaR0w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='VkmKLUCaMsYFCuGE0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_mCherry_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='A-MzQvq-kHeu1WHdVmm5bw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='h4EKWveW36LIzXez0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_Alexa488_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='ZPUWq_TI3KWNcVeVz3vOPg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='fdem35nw5ztUnEIM0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_DAPI_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='XuugTTV9S5TbJKVMLOaR0w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='VkmKLUCaMsYFCuGE0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_mCherry_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='A-MzQvq-kHeu1WHdVmm5bw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='ThuJnRAhqkp54kyU0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_Alexa488_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='vs3t8d40w259zgJRltHlKA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='PquYNyshQTDd24Vw0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_DAPI_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='1mSZ_MMg393bfTvMOl3YMQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='8SFUmW0RhBNySxBO0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_mCherry_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='fjO8ly0euUPHXoQd86weww', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='ThuJnRAhqkp54kyU0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_Alexa488_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='vs3t8d40w259zgJRltHlKA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='PquYNyshQTDd24Vw0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_DAPI_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='1mSZ_MMg393bfTvMOl3YMQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='8SFUmW0RhBNySxBO0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_mCherry_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='fjO8ly0euUPHXoQd86weww', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='h4EKWveW36LIzXez0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_Alexa488_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='ZPUWq_TI3KWNcVeVz3vOPg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='fdem35nw5ztUnEIM0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_DAPI_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='XuugTTV9S5TbJKVMLOaR0w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='VkmKLUCaMsYFCuGE0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_mCherry_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='A-MzQvq-kHeu1WHdVmm5bw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='h4EKWveW36LIzXez0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_Alexa488_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='ZPUWq_TI3KWNcVeVz3vOPg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='fdem35nw5ztUnEIM0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_DAPI_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='XuugTTV9S5TbJKVMLOaR0w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='VkmKLUCaMsYFCuGE0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_mCherry_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='A-MzQvq-kHeu1WHdVmm5bw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='QDPX1ljp0eCMz80o0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_Alexa488_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='Y1bgVRJGzIEhKFGP54fplA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='Cvamog4G3a2XYGM80000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_DAPI_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='UFjAP7uBiaM9ivE4hJzHTA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='6uUjyphUD4D1Hixc0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_mCherry_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='lvFoCpbAkjg4mEtXx-D99w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='QDPX1ljp0eCMz80o0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_Alexa488_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='Y1bgVRJGzIEhKFGP54fplA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='Cvamog4G3a2XYGM80000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_DAPI_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='UFjAP7uBiaM9ivE4hJzHTA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='6uUjyphUD4D1Hixc0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_mCherry_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='lvFoCpbAkjg4mEtXx-D99w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Oww4y0yYuR8pxV9q0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_Alexa488_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='YX1aIPYndKFiPl5W3OPqfQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='AhBvnNKg5yJcG6LU0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_DAPI_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9I6amjQc298AZvER1Z2jjQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='AVRTVX9gEu4LrTAP0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_mCherry_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='rYoK8xKXBtx5aNW4AfUbUg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Oww4y0yYuR8pxV9q0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_Alexa488_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='YX1aIPYndKFiPl5W3OPqfQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='AhBvnNKg5yJcG6LU0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_DAPI_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9I6amjQc298AZvER1Z2jjQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='AVRTVX9gEu4LrTAP0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_mCherry_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='rYoK8xKXBtx5aNW4AfUbUg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='QDPX1ljp0eCMz80o0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_Alexa488_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='Y1bgVRJGzIEhKFGP54fplA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='Cvamog4G3a2XYGM80000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_DAPI_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='UFjAP7uBiaM9ivE4hJzHTA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='6uUjyphUD4D1Hixc0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_mCherry_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='lvFoCpbAkjg4mEtXx-D99w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='QDPX1ljp0eCMz80o0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_Alexa488_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='Y1bgVRJGzIEhKFGP54fplA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='Cvamog4G3a2XYGM80000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_DAPI_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='UFjAP7uBiaM9ivE4hJzHTA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='6uUjyphUD4D1Hixc0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_mCherry_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='lvFoCpbAkjg4mEtXx-D99w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Oww4y0yYuR8pxV9q0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_Alexa488_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='YX1aIPYndKFiPl5W3OPqfQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='AhBvnNKg5yJcG6LU0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_DAPI_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9I6amjQc298AZvER1Z2jjQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='AVRTVX9gEu4LrTAP0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_mCherry_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='rYoK8xKXBtx5aNW4AfUbUg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Oww4y0yYuR8pxV9q0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_Alexa488_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='YX1aIPYndKFiPl5W3OPqfQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='AhBvnNKg5yJcG6LU0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_DAPI_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9I6amjQc298AZvER1Z2jjQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='AVRTVX9gEu4LrTAP0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_mCherry_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='rYoK8xKXBtx5aNW4AfUbUg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Gtwi9Pcyx8maQEWB0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_Alexa488_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='yJMlFFYyup0JmBu_FjTa3g', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='sHHpiiFYWsIXMZNV0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_DAPI_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='6pvuFREF3CQ8xr8Urak52Q', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='hNMkrIHce1XrLZHY0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_mCherry_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='K1jGiIE0dljR_qYP1btlyw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Gtwi9Pcyx8maQEWB0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_Alexa488_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='yJMlFFYyup0JmBu_FjTa3g', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='sHHpiiFYWsIXMZNV0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_DAPI_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='6pvuFREF3CQ8xr8Urak52Q', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='hNMkrIHce1XrLZHY0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_mCherry_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='K1jGiIE0dljR_qYP1btlyw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='nSZhAypqiNZ2Ylbe0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_Alexa488_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='uh537K7rMhMNKW-mti1vjw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='jzqxtoduIJ3hCbB40000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_DAPI_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='SOxM7EH80KPXK3hzBxXYLg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='M06liaIzh2OVEuJ40000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_mCherry_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='0tV4uQYxnP6G8JOrAwMyXw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='nSZhAypqiNZ2Ylbe0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_Alexa488_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='uh537K7rMhMNKW-mti1vjw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='jzqxtoduIJ3hCbB40000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_DAPI_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='SOxM7EH80KPXK3hzBxXYLg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='M06liaIzh2OVEuJ40000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_mCherry_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='0tV4uQYxnP6G8JOrAwMyXw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Gtwi9Pcyx8maQEWB0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_Alexa488_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='yJMlFFYyup0JmBu_FjTa3g', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='sHHpiiFYWsIXMZNV0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_DAPI_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='6pvuFREF3CQ8xr8Urak52Q', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='hNMkrIHce1XrLZHY0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_mCherry_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='K1jGiIE0dljR_qYP1btlyw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Gtwi9Pcyx8maQEWB0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_Alexa488_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='yJMlFFYyup0JmBu_FjTa3g', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='sHHpiiFYWsIXMZNV0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_DAPI_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='6pvuFREF3CQ8xr8Urak52Q', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='hNMkrIHce1XrLZHY0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_mCherry_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='K1jGiIE0dljR_qYP1btlyw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='nSZhAypqiNZ2Ylbe0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_Alexa488_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='uh537K7rMhMNKW-mti1vjw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='jzqxtoduIJ3hCbB40000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_DAPI_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='SOxM7EH80KPXK3hzBxXYLg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='M06liaIzh2OVEuJ40000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_mCherry_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='0tV4uQYxnP6G8JOrAwMyXw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='nSZhAypqiNZ2Ylbe0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_Alexa488_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='uh537K7rMhMNKW-mti1vjw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='jzqxtoduIJ3hCbB40000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_DAPI_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='SOxM7EH80KPXK3hzBxXYLg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='M06liaIzh2OVEuJ40000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row01_Well08_mCherry_zstack001_r002_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='0tV4uQYxnP6G8JOrAwMyXw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='mXWwV1x42Jz9RoSO0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_Alexa488_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='4xc4bpAMo0UaM_tc8SciIA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='gj0HHnoVpEqbaUJb0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_DAPI_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='fZ4W2ePHagfGUGCcve98gQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='1XnEyqVt6UGXCTmV0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_mCherry_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='if3WCKH91_CY0ptIVYuLmA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='mXWwV1x42Jz9RoSO0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_Alexa488_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='4xc4bpAMo0UaM_tc8SciIA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='gj0HHnoVpEqbaUJb0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_DAPI_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='fZ4W2ePHagfGUGCcve98gQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='1XnEyqVt6UGXCTmV0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_mCherry_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='if3WCKH91_CY0ptIVYuLmA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Ov8FnKzHMNY0XVJa0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_Alexa488_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='5cfc02GejBaoJ0h3OLWSdw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='Lwk8shsYe0V5bMgd0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_DAPI_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='CmAa139DH_lQ2Plcy4BxXw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='YuyVn060M4FxATPz0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_mCherry_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='gZHwk76xPz7qXiST4RuVMQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Ov8FnKzHMNY0XVJa0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_Alexa488_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='5cfc02GejBaoJ0h3OLWSdw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='Lwk8shsYe0V5bMgd0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_DAPI_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='CmAa139DH_lQ2Plcy4BxXw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='YuyVn060M4FxATPz0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_mCherry_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='gZHwk76xPz7qXiST4RuVMQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='mXWwV1x42Jz9RoSO0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_Alexa488_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='4xc4bpAMo0UaM_tc8SciIA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='gj0HHnoVpEqbaUJb0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_DAPI_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='fZ4W2ePHagfGUGCcve98gQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='1XnEyqVt6UGXCTmV0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_mCherry_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='if3WCKH91_CY0ptIVYuLmA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='mXWwV1x42Jz9RoSO0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_Alexa488_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='4xc4bpAMo0UaM_tc8SciIA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='gj0HHnoVpEqbaUJb0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_DAPI_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='fZ4W2ePHagfGUGCcve98gQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='1XnEyqVt6UGXCTmV0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_mCherry_zstack001_r002_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='if3WCKH91_CY0ptIVYuLmA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Ov8FnKzHMNY0XVJa0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_Alexa488_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='5cfc02GejBaoJ0h3OLWSdw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='Lwk8shsYe0V5bMgd0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_DAPI_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='CmAa139DH_lQ2Plcy4BxXw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='YuyVn060M4FxATPz0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_mCherry_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='gZHwk76xPz7qXiST4RuVMQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Ov8FnKzHMNY0XVJa0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_Alexa488_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='5cfc02GejBaoJ0h3OLWSdw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='Lwk8shsYe0V5bMgd0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_DAPI_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='CmAa139DH_lQ2Plcy4BxXw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='YuyVn060M4FxATPz0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row02_Well08_mCherry_zstack001_r002_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='gZHwk76xPz7qXiST4RuVMQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='ThuJnRAhqkp54kyU0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_Alexa488_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='vs3t8d40w259zgJRltHlKA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='PquYNyshQTDd24Vw0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_DAPI_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='1mSZ_MMg393bfTvMOl3YMQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='8SFUmW0RhBNySxBO0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_mCherry_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='fjO8ly0euUPHXoQd86weww', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='ThuJnRAhqkp54kyU0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_Alexa488_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='vs3t8d40w259zgJRltHlKA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='PquYNyshQTDd24Vw0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_DAPI_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='1mSZ_MMg393bfTvMOl3YMQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='8SFUmW0RhBNySxBO0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_mCherry_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='fjO8ly0euUPHXoQd86weww', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='h4EKWveW36LIzXez0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_Alexa488_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='ZPUWq_TI3KWNcVeVz3vOPg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='fdem35nw5ztUnEIM0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_DAPI_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='XuugTTV9S5TbJKVMLOaR0w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='VkmKLUCaMsYFCuGE0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_mCherry_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='A-MzQvq-kHeu1WHdVmm5bw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='h4EKWveW36LIzXez0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_Alexa488_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='ZPUWq_TI3KWNcVeVz3vOPg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='fdem35nw5ztUnEIM0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_DAPI_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='XuugTTV9S5TbJKVMLOaR0w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='VkmKLUCaMsYFCuGE0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_mCherry_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='A-MzQvq-kHeu1WHdVmm5bw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='ThuJnRAhqkp54kyU0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_Alexa488_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='vs3t8d40w259zgJRltHlKA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='PquYNyshQTDd24Vw0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_DAPI_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='1mSZ_MMg393bfTvMOl3YMQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='8SFUmW0RhBNySxBO0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_mCherry_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='fjO8ly0euUPHXoQd86weww', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='ThuJnRAhqkp54kyU0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_Alexa488_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='vs3t8d40w259zgJRltHlKA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='PquYNyshQTDd24Vw0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_DAPI_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='1mSZ_MMg393bfTvMOl3YMQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='8SFUmW0RhBNySxBO0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_mCherry_zstack001_r010_c009.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='fjO8ly0euUPHXoQd86weww', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='h4EKWveW36LIzXez0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_Alexa488_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='ZPUWq_TI3KWNcVeVz3vOPg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='fdem35nw5ztUnEIM0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_DAPI_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='XuugTTV9S5TbJKVMLOaR0w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='VkmKLUCaMsYFCuGE0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_mCherry_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='A-MzQvq-kHeu1WHdVmm5bw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='h4EKWveW36LIzXez0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_Alexa488_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='ZPUWq_TI3KWNcVeVz3vOPg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='fdem35nw5ztUnEIM0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_DAPI_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='XuugTTV9S5TbJKVMLOaR0w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='VkmKLUCaMsYFCuGE0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row03_Well08_mCherry_zstack001_r010_c010.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='A-MzQvq-kHeu1WHdVmm5bw', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='QDPX1ljp0eCMz80o0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_Alexa488_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='Y1bgVRJGzIEhKFGP54fplA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='Cvamog4G3a2XYGM80000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_DAPI_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='UFjAP7uBiaM9ivE4hJzHTA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='6uUjyphUD4D1Hixc0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_mCherry_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='lvFoCpbAkjg4mEtXx-D99w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='QDPX1ljp0eCMz80o0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_Alexa488_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='Y1bgVRJGzIEhKFGP54fplA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='Cvamog4G3a2XYGM80000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_DAPI_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='UFjAP7uBiaM9ivE4hJzHTA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='6uUjyphUD4D1Hixc0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_mCherry_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='lvFoCpbAkjg4mEtXx-D99w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Oww4y0yYuR8pxV9q0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_Alexa488_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='YX1aIPYndKFiPl5W3OPqfQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='AhBvnNKg5yJcG6LU0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_DAPI_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9I6amjQc298AZvER1Z2jjQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='AVRTVX9gEu4LrTAP0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_mCherry_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='rYoK8xKXBtx5aNW4AfUbUg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Oww4y0yYuR8pxV9q0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_Alexa488_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='YX1aIPYndKFiPl5W3OPqfQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='AhBvnNKg5yJcG6LU0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_DAPI_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9I6amjQc298AZvER1Z2jjQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='AVRTVX9gEu4LrTAP0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_mCherry_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='rYoK8xKXBtx5aNW4AfUbUg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='QDPX1ljp0eCMz80o0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_Alexa488_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='Y1bgVRJGzIEhKFGP54fplA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='Cvamog4G3a2XYGM80000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_DAPI_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='UFjAP7uBiaM9ivE4hJzHTA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='6uUjyphUD4D1Hixc0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_mCherry_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='lvFoCpbAkjg4mEtXx-D99w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='QDPX1ljp0eCMz80o0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_Alexa488_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='Y1bgVRJGzIEhKFGP54fplA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:24 UTC), Artifact(uid='Cvamog4G3a2XYGM80000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_DAPI_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='UFjAP7uBiaM9ivE4hJzHTA', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='6uUjyphUD4D1Hixc0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_mCherry_zstack001_r001_c003.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='lvFoCpbAkjg4mEtXx-D99w', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Oww4y0yYuR8pxV9q0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_Alexa488_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='YX1aIPYndKFiPl5W3OPqfQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='AhBvnNKg5yJcG6LU0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_DAPI_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9I6amjQc298AZvER1Z2jjQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='AVRTVX9gEu4LrTAP0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_mCherry_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='rYoK8xKXBtx5aNW4AfUbUg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
! cannot infer feature type of: <QuerySet [Artifact(uid='Oww4y0yYuR8pxV9q0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_Alexa488_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='YX1aIPYndKFiPl5W3OPqfQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='AhBvnNKg5yJcG6LU0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_DAPI_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='9I6amjQc298AZvER1Z2jjQ', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC), Artifact(uid='AVRTVX9gEu4LrTAP0000', is_latest=True, key='input_data_imaging_usecase/images/Timepoint001_Row04_Well08_mCherry_zstack001_r001_c005.tif', description='raw image of U2OS cells stained for autophagy markers', suffix='.tif', size=2333056, hash='rYoK8xKXBtx5aNW4AfUbUg', space_id=1, storage_id=2, run_id=2, created_by_id=1, created_at=2025-03-07 13:51:25 UTC)]>, returning '?
Dataset already processed and results uploaded to instance. Skipping processing.
example_artifact = ln.Artifact.filter(
ulabels=ln.ULabel.get(name="scportrait single-cell images")
)[0]
example_artifact.view_lineage()
ln.finish()
Show code cell output
→ finished Run('5F6RMbrE') after 4m at 2025-03-31 12:50:16 UTC