sc-imaging¶
Here, you will learn how to structure, featurize, and make a large imaging collection queryable for large-scale machine learning:
Load and annotate a
Collection
of microscopy images ()
First, we load and annotate a collection of microscopy images in TIFF format that was previously uploaded.
The images used here were acquired as part of a study on autophagy, a cellular process during which cells recycle their components in autophagosomes. The study tracked genetic determinants of autophagy through fluorescence microscopy of human U2OS cells.
# pip install 'lamindb[jupyter,bionty]'
!lamin init --storage ./test-sc-imaging --modules bionty
Show code cell output
→ initialized lamindb: testuser1/test-sc-imaging
import lamindb as ln
import bionty as bt
from tifffile import imread
import matplotlib.pyplot as plt
ln.track()
Show code cell output
→ connected lamindb: testuser1/test-sc-imaging
→ created Transform('bYTBim7BjUCL0000'), started new Run('4TQVxxtn...') at 2025-06-03 16:30:43 UTC
→ notebook imports: bionty==1.5.0 lamindb==1.6.1 matplotlib==3.10.3 tifffile==2025.6.1
• recommendation: to identify the notebook across renames, pass the uid: ln.track("bYTBim7BjUCL")
All image metadata is stored in an already ingested .csv
file on the scportrait/examples
instance.
metadata_files = (
ln.Artifact.using("scportrait/examples")
.get(key="input_data_imaging_usecase/metadata_files.csv")
.load()
)
metadata_files.head(2)
Show code cell output
→ transferred records: Artifact(uid='jdjwcUB0w1QAHAYm0000'), Storage(uid='r7YUayXjktSb')
image_path | genotype | stimulation | cell_line | cell_line_clone | channel | FOV | magnification | microscope | imaged structure | resolution | |
---|---|---|---|---|---|---|---|---|---|---|---|
0 | input_data_imaging_usecase/images/Timepoint001... | WT | 14h Torin-1 | U2OS | U2OS lcklip-mNeon mCherryLC3B clone 1 | Alexa488 | FOV1 | 20X | Opera Phenix | LckLip-mNeon | 0.597976 |
1 | input_data_imaging_usecase/images/Timepoint001... | WT | 14h Torin-1 | U2OS | U2OS lcklip-mNeon mCherryLC3B clone 1 | Alexa488 | FOV2 | 20X | Opera Phenix | LckLip-mNeon | 0.597976 |
metadata_files.apply(lambda col: col.unique())
Show code cell output
image_path [input_data_imaging_usecase/images/Timepoint00...
genotype [WT, EI24KO]
stimulation [14h Torin-1, untreated]
cell_line [U2OS]
cell_line_clone [U2OS lcklip-mNeon mCherryLC3B clone 1, U2OS l...
channel [Alexa488, DAPI, mCherry]
FOV [FOV1, FOV2]
magnification [20X]
microscope [Opera Phenix]
imaged structure [LckLip-mNeon, DNA, mCherry-LC3B]
resolution [0.597976081]
dtype: object
All images are of the same cell line (U2OS), which have been imaged on an Opera Phenix microscope at 20X magnification.
To induce autophagy, the cells have either been treated with Torin-1
, a small molecule that mimics starvation, for 14 hours, or left untreated as a control.
To visualize the process of autophagosome formation, the U2OS cells have been genetically engineered to express fluorescently tagged proteins.
LC3B
is a marker of autophagosomes, allowing us to visualize their formation in the mCherry channel.
LckLip
is a membrane-targeted fluorescent protein, which helps outline the cellular boundaries of individual cells in the Alexa488
channel.
Furthermore, the cells’ DNA was stained using Hoechst
, which we can visualize in the DAPI
channel to identify the nuclei of individual cells.
These three structures are visualized in three separate image channels:
Channel |
Imaged Structure |
---|---|
1 |
DNA |
2 |
Autophagosomes |
3 |
Plasma Membrane |
In addition to expressing fluorescently tagged proteins, some of the cells have had the EI24
gene knocked out, leading to two different genotypes: WT
(wild-type) cells and EI24KO
(knockout) cells.
For each genotype, two different clonal cell lines were analyzed, with multiple fields of view (FOVs) captured per condition.
To enable queries on our images, we annotate them with the corresponding metadata.
autophagy_imaging_schema = ln.Schema(
name="Autophagy imaging schema",
features=[
ln.Feature(name="genotype", dtype=ln.ULabel.name).save(),
ln.Feature(name="stimulation", dtype=ln.ULabel.name).save(),
ln.Feature(name="cell_line", dtype=bt.CellLine.name).save(),
ln.Feature(name="cell_line_clone", dtype=ln.ULabel.name).save(),
ln.Feature(name="channel", dtype=ln.ULabel.name).save(),
ln.Feature(name="FOV", dtype=ln.ULabel.name).save(),
ln.Feature(name="magnification", dtype=ln.ULabel.name).save(),
ln.Feature(name="microscope", dtype=ln.ULabel.name).save(),
ln.Feature(name="imaged structure", dtype=ln.ULabel.name).save(),
ln.Feature(
name="resolution", dtype=float, description="conversion factor for px to µm"
).save(),
],
coerce_dtype=True,
).save()
curator = ln.curators.DataFrameCurator(metadata_files, autophagy_imaging_schema)
try:
curator.validate()
except ln.core.exceptions.ValidationError as e:
print(e)
Show code cell output
! 2 terms not validated in feature 'genotype': 'WT', 'EI24KO'
→ fix typos, remove non-existent values, or save terms via: curator.cat.add_new_from('genotype')
! 2 terms not validated in feature 'stimulation': '14h Torin-1', 'untreated'
→ fix typos, remove non-existent values, or save terms via: curator.cat.add_new_from('stimulation')
! 1 term not validated in feature 'cell_line': 'U2OS'
1 synonym found: "U2OS" → "U-2 OS cell"
→ curate synonyms via: .standardize("cell_line")
! 4 terms not validated in feature 'cell_line_clone': 'U2OS lcklip-mNeon mCherryLC3B clone 1', 'U2OS lcklip-mNeon mCherryLC3B clone 2', 'U2OS lcklip-mNeon mCherryLC3B EI24 KO clone 1', 'U2OS lcklip-mNeon mCherryLC3B EI24 KO clone 2'
→ fix typos, remove non-existent values, or save terms via: curator.cat.add_new_from('cell_line_clone')
! 3 terms not validated in feature 'channel': 'Alexa488', 'DAPI', 'mCherry'
→ fix typos, remove non-existent values, or save terms via: curator.cat.add_new_from('channel')
! 2 terms not validated in feature 'FOV': 'FOV1', 'FOV2'
→ fix typos, remove non-existent values, or save terms via: curator.cat.add_new_from('FOV')
! 1 term not validated in feature 'magnification': '20X'
→ fix typos, remove non-existent values, or save terms via: curator.cat.add_new_from('magnification')
! 1 term not validated in feature 'microscope': 'Opera Phenix'
→ fix typos, remove non-existent values, or save terms via: curator.cat.add_new_from('microscope')
! 3 terms not validated in feature 'imaged structure': 'LckLip-mNeon', 'DNA', 'mCherry-LC3B'
→ fix typos, remove non-existent values, or save terms via: curator.cat.add_new_from('imaged structure')
3 terms not validated in feature 'imaged structure': 'LckLip-mNeon', 'DNA', 'mCherry-LC3B'
→ fix typos, remove non-existent values, or save terms via: curator.cat.add_new_from('imaged structure')
Add and standardize missing terms:
curator.cat.standardize("cell_line")
curator.cat.add_new_from("all")
curator.validate()
Show code cell output
! 'all' is deprecated, please pass a single key from `.non_validated.keys()` instead!
! records with similar names exist! did you mean to load one of them?
uid | name | is_type | description | reference | reference_type | space_id | type_id | run_id | created_at | created_by_id | _aux | branch_id | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
id | |||||||||||||
5 | 9EAQMWeF | U2OS lcklip-mNeon mCherryLC3B clone 1 | False | None | None | None | 1 | None | 1 | 2025-06-03 16:30:51.683000+00:00 | 1 | None | 1 |
6 | p1R4W92j | U2OS lcklip-mNeon mCherryLC3B clone 2 | False | None | None | None | 1 | None | 1 | 2025-06-03 16:30:51.683000+00:00 | 1 | None | 1 |
7 | ilBy8ry6 | U2OS lcklip-mNeon mCherryLC3B EI24 KO clone 1 | False | None | None | None | 1 | None | 1 | 2025-06-03 16:30:51.683000+00:00 | 1 | None | 1 |
! records with similar names exist! did you mean to load one of them?
uid | name | is_type | description | reference | reference_type | space_id | type_id | run_id | created_at | created_by_id | _aux | branch_id | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
id | |||||||||||||
5 | 9EAQMWeF | U2OS lcklip-mNeon mCherryLC3B clone 1 | False | None | None | None | 1 | None | 1 | 2025-06-03 16:30:51.683000+00:00 | 1 | None | 1 |
6 | p1R4W92j | U2OS lcklip-mNeon mCherryLC3B clone 2 | False | None | None | None | 1 | None | 1 | 2025-06-03 16:30:51.683000+00:00 | 1 | None | 1 |
7 | ilBy8ry6 | U2OS lcklip-mNeon mCherryLC3B EI24 KO clone 1 | False | None | None | None | 1 | None | 1 | 2025-06-03 16:30:51.683000+00:00 | 1 | None | 1 |
Add all images to our lamindb instance to annotate all relevant metadata.
# Create study feature and associated label
ln.Feature(name="study", dtype=ln.ULabel).save()
ln.ULabel(name="autophagy imaging").save()
# loop through all Artifacts and add feature values
artifacts = []
for _, row in metadata_files.iterrows():
artifact = (
ln.Artifact.using("scportrait/examples")
.filter(key__icontains=row["image_path"])
.one()
)
artifact.save()
artifact.cell_lines.add(bt.CellLine.filter(name=row.cell_line).one())
artifact.features.add_values(
{
"genotype": row.genotype,
"stimulation": row.stimulation,
"cell_line_clone": row.cell_line_clone,
"channel": row.channel,
"imaged structure": row["imaged structure"],
"study": "autophagy imaging",
"FOV": row.FOV,
"magnification": row.magnification,
"microscope": row.microscope,
"resolution": row.resolution,
}
)
artifacts.append(artifact)
Show code cell output
→ transferred records: Artifact(uid='Md4OouMExlWS2YfZ0000'), CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='A2945i5P'), ULabel(uid='KH1brNUW'), ULabel(uid='XKI9Mx33'), ULabel(uid='CrR7fgIZ'), ULabel(uid='QrU6fxsG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='A2945i5P'), ULabel(uid='KH1brNUW'), ULabel(uid='XKI9Mx33'), ULabel(uid='QrU6fxsG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='KKbVRkOjQ1jdA2fx0000'), ULabel(uid='1KPobNqK')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='A2945i5P'), ULabel(uid='KH1brNUW'), ULabel(uid='CrR7fgIZ'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='CiQYTBNZrj0CPejK0000'), ULabel(uid='HK8F1lVS'), ULabel(uid='xhpmj7p7')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='HK8F1lVS'), ULabel(uid='9hHptuyb'), ULabel(uid='A2945i5P'), ULabel(uid='KH1brNUW'), ULabel(uid='1KPobNqK'), ULabel(uid='xhpmj7p7'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='W6tzE7JNiM80Ruho0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='A2945i5P'), ULabel(uid='KH1brNUW'), ULabel(uid='CrR7fgIZ'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='YGiNq6DPfIEjtt9j0000'), ULabel(uid='AyafKoQw'), ULabel(uid='xHqZKcIG')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='A2945i5P'), ULabel(uid='KH1brNUW'), ULabel(uid='AyafKoQw'), ULabel(uid='1KPobNqK'), ULabel(uid='xHqZKcIG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='uuh41FAHEz0ASL2N0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='KH1brNUW'), ULabel(uid='XKI9Mx33'), ULabel(uid='CrR7fgIZ'), ULabel(uid='QrU6fxsG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='Gtwi9Pcyx8maQEWB0000'), ULabel(uid='EgihgpJC')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='EgihgpJC'), ULabel(uid='KH1brNUW'), ULabel(uid='XKI9Mx33'), ULabel(uid='1KPobNqK'), ULabel(uid='QrU6fxsG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='nSZhAypqiNZ2Ylbe0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='HK8F1lVS'), ULabel(uid='9hHptuyb'), ULabel(uid='EgihgpJC'), ULabel(uid='KH1brNUW'), ULabel(uid='CrR7fgIZ'), ULabel(uid='xhpmj7p7'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='sHHpiiFYWsIXMZNV0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='HK8F1lVS'), ULabel(uid='9hHptuyb'), ULabel(uid='EgihgpJC'), ULabel(uid='KH1brNUW'), ULabel(uid='1KPobNqK'), ULabel(uid='xhpmj7p7'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='jzqxtoduIJ3hCbB40000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='EgihgpJC'), ULabel(uid='KH1brNUW'), ULabel(uid='AyafKoQw'), ULabel(uid='CrR7fgIZ'), ULabel(uid='xHqZKcIG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='hNMkrIHce1XrLZHY0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='EgihgpJC'), ULabel(uid='KH1brNUW'), ULabel(uid='AyafKoQw'), ULabel(uid='1KPobNqK'), ULabel(uid='xHqZKcIG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='M06liaIzh2OVEuJ40000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='A2945i5P'), ULabel(uid='XKI9Mx33'), ULabel(uid='CrR7fgIZ'), ULabel(uid='QrU6fxsG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='yiIMSAddDWgLgki70000'), ULabel(uid='GadMJgvN')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='A2945i5P'), ULabel(uid='GadMJgvN'), ULabel(uid='XKI9Mx33'), ULabel(uid='1KPobNqK'), ULabel(uid='QrU6fxsG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='2ie2Kjzn1O7UYhuq0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='HK8F1lVS'), ULabel(uid='9hHptuyb'), ULabel(uid='A2945i5P'), ULabel(uid='GadMJgvN'), ULabel(uid='CrR7fgIZ'), ULabel(uid='xhpmj7p7'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='DEzw4QQAsjVZ010b0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='HK8F1lVS'), ULabel(uid='9hHptuyb'), ULabel(uid='A2945i5P'), ULabel(uid='GadMJgvN'), ULabel(uid='1KPobNqK'), ULabel(uid='xhpmj7p7'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='fOQSb7JCK67aeN6a0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='A2945i5P'), ULabel(uid='GadMJgvN'), ULabel(uid='AyafKoQw'), ULabel(uid='CrR7fgIZ'), ULabel(uid='xHqZKcIG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='hbVyCGFARHU91Kax0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='A2945i5P'), ULabel(uid='GadMJgvN'), ULabel(uid='AyafKoQw'), ULabel(uid='1KPobNqK'), ULabel(uid='xHqZKcIG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='qHQpdWcFu7FzF6l50000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='EgihgpJC'), ULabel(uid='GadMJgvN'), ULabel(uid='XKI9Mx33'), ULabel(uid='CrR7fgIZ'), ULabel(uid='QrU6fxsG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='mXWwV1x42Jz9RoSO0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='EgihgpJC'), ULabel(uid='GadMJgvN'), ULabel(uid='XKI9Mx33'), ULabel(uid='1KPobNqK'), ULabel(uid='QrU6fxsG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='Ov8FnKzHMNY0XVJa0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='HK8F1lVS'), ULabel(uid='9hHptuyb'), ULabel(uid='EgihgpJC'), ULabel(uid='GadMJgvN'), ULabel(uid='CrR7fgIZ'), ULabel(uid='xhpmj7p7'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='gj0HHnoVpEqbaUJb0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='HK8F1lVS'), ULabel(uid='9hHptuyb'), ULabel(uid='EgihgpJC'), ULabel(uid='GadMJgvN'), ULabel(uid='1KPobNqK'), ULabel(uid='xhpmj7p7'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='Lwk8shsYe0V5bMgd0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='EgihgpJC'), ULabel(uid='GadMJgvN'), ULabel(uid='AyafKoQw'), ULabel(uid='CrR7fgIZ'), ULabel(uid='xHqZKcIG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='1XnEyqVt6UGXCTmV0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='EgihgpJC'), ULabel(uid='GadMJgvN'), ULabel(uid='AyafKoQw'), ULabel(uid='1KPobNqK'), ULabel(uid='xHqZKcIG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='YuyVn060M4FxATPz0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='A2945i5P'), ULabel(uid='XKI9Mx33'), ULabel(uid='CrR7fgIZ'), ULabel(uid='QrU6fxsG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='jVytS8AyAHmHkYR30000'), ULabel(uid='Aj8KGwbh'), ULabel(uid='JIbWVXma')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='Aj8KGwbh'), ULabel(uid='A2945i5P'), ULabel(uid='JIbWVXma'), ULabel(uid='XKI9Mx33'), ULabel(uid='1KPobNqK'), ULabel(uid='QrU6fxsG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='vCVbKkzz4CnJPPKF0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='HK8F1lVS'), ULabel(uid='Aj8KGwbh'), ULabel(uid='A2945i5P'), ULabel(uid='JIbWVXma'), ULabel(uid='CrR7fgIZ'), ULabel(uid='xhpmj7p7'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='cw4F6bUB9zuMthCY0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='HK8F1lVS'), ULabel(uid='Aj8KGwbh'), ULabel(uid='A2945i5P'), ULabel(uid='JIbWVXma'), ULabel(uid='1KPobNqK'), ULabel(uid='xhpmj7p7'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='5kMhlcDNek4RMeQF0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='Aj8KGwbh'), ULabel(uid='A2945i5P'), ULabel(uid='JIbWVXma'), ULabel(uid='AyafKoQw'), ULabel(uid='CrR7fgIZ'), ULabel(uid='xHqZKcIG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='7TZGXvbA0JLL68hR0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='Aj8KGwbh'), ULabel(uid='A2945i5P'), ULabel(uid='JIbWVXma'), ULabel(uid='AyafKoQw'), ULabel(uid='1KPobNqK'), ULabel(uid='xHqZKcIG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='9BmbViqMmlVhpfS00000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='Aj8KGwbh'), ULabel(uid='EgihgpJC'), ULabel(uid='JIbWVXma'), ULabel(uid='XKI9Mx33'), ULabel(uid='CrR7fgIZ'), ULabel(uid='QrU6fxsG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='ThuJnRAhqkp54kyU0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='Aj8KGwbh'), ULabel(uid='EgihgpJC'), ULabel(uid='JIbWVXma'), ULabel(uid='XKI9Mx33'), ULabel(uid='1KPobNqK'), ULabel(uid='QrU6fxsG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='h4EKWveW36LIzXez0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='HK8F1lVS'), ULabel(uid='Aj8KGwbh'), ULabel(uid='EgihgpJC'), ULabel(uid='JIbWVXma'), ULabel(uid='CrR7fgIZ'), ULabel(uid='xhpmj7p7'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='PquYNyshQTDd24Vw0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='HK8F1lVS'), ULabel(uid='Aj8KGwbh'), ULabel(uid='EgihgpJC'), ULabel(uid='JIbWVXma'), ULabel(uid='1KPobNqK'), ULabel(uid='xhpmj7p7'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='fdem35nw5ztUnEIM0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='Aj8KGwbh'), ULabel(uid='EgihgpJC'), ULabel(uid='JIbWVXma'), ULabel(uid='AyafKoQw'), ULabel(uid='CrR7fgIZ'), ULabel(uid='xHqZKcIG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='8SFUmW0RhBNySxBO0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='Aj8KGwbh'), ULabel(uid='EgihgpJC'), ULabel(uid='JIbWVXma'), ULabel(uid='AyafKoQw'), ULabel(uid='1KPobNqK'), ULabel(uid='xHqZKcIG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='VkmKLUCaMsYFCuGE0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='Aj8KGwbh'), ULabel(uid='A2945i5P'), ULabel(uid='XKI9Mx33'), ULabel(uid='CrR7fgIZ'), ULabel(uid='QrU6fxsG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='OS0wBE7bviIlW7qj0000'), ULabel(uid='joRCMMWX')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='Aj8KGwbh'), ULabel(uid='A2945i5P'), ULabel(uid='joRCMMWX'), ULabel(uid='XKI9Mx33'), ULabel(uid='1KPobNqK'), ULabel(uid='QrU6fxsG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='9ZVngbl0JUS0XdZ70000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='HK8F1lVS'), ULabel(uid='Aj8KGwbh'), ULabel(uid='A2945i5P'), ULabel(uid='joRCMMWX'), ULabel(uid='CrR7fgIZ'), ULabel(uid='xhpmj7p7'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='ixOpuSTsyrPXdYuA0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='HK8F1lVS'), ULabel(uid='Aj8KGwbh'), ULabel(uid='A2945i5P'), ULabel(uid='joRCMMWX'), ULabel(uid='1KPobNqK'), ULabel(uid='xhpmj7p7'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='IzP3IAwIhmM7OORD0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='Aj8KGwbh'), ULabel(uid='A2945i5P'), ULabel(uid='joRCMMWX'), ULabel(uid='AyafKoQw'), ULabel(uid='CrR7fgIZ'), ULabel(uid='xHqZKcIG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='6uMjKAk1aYlAV7Cf0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='Aj8KGwbh'), ULabel(uid='A2945i5P'), ULabel(uid='joRCMMWX'), ULabel(uid='AyafKoQw'), ULabel(uid='1KPobNqK'), ULabel(uid='xHqZKcIG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='RRVS8qVx3VSw02Xu0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='Aj8KGwbh'), ULabel(uid='EgihgpJC'), ULabel(uid='joRCMMWX'), ULabel(uid='XKI9Mx33'), ULabel(uid='CrR7fgIZ'), ULabel(uid='QrU6fxsG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='QDPX1ljp0eCMz80o0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='Aj8KGwbh'), ULabel(uid='EgihgpJC'), ULabel(uid='joRCMMWX'), ULabel(uid='XKI9Mx33'), ULabel(uid='1KPobNqK'), ULabel(uid='QrU6fxsG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='Oww4y0yYuR8pxV9q0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='HK8F1lVS'), ULabel(uid='Aj8KGwbh'), ULabel(uid='EgihgpJC'), ULabel(uid='joRCMMWX'), ULabel(uid='CrR7fgIZ'), ULabel(uid='xhpmj7p7'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='Cvamog4G3a2XYGM80000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='HK8F1lVS'), ULabel(uid='Aj8KGwbh'), ULabel(uid='EgihgpJC'), ULabel(uid='joRCMMWX'), ULabel(uid='1KPobNqK'), ULabel(uid='xhpmj7p7'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='AhBvnNKg5yJcG6LU0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='Aj8KGwbh'), ULabel(uid='EgihgpJC'), ULabel(uid='joRCMMWX'), ULabel(uid='AyafKoQw'), ULabel(uid='CrR7fgIZ'), ULabel(uid='xHqZKcIG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='6uUjyphUD4D1Hixc0000')
→ mapped records: CellLine(uid='4oLoqaUP'), ULabel(uid='Aj8KGwbh'), ULabel(uid='EgihgpJC'), ULabel(uid='joRCMMWX'), ULabel(uid='AyafKoQw'), ULabel(uid='1KPobNqK'), ULabel(uid='xHqZKcIG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ transferred records: Artifact(uid='AVRTVX9gEu4LrTAP0000')
artifacts[0].describe()
Artifact .tif ├── General │ ├── .uid = 'Md4OouMExlWS2YfZ0000' │ ├── .key = 'input_data_imaging_usecase/images/Timepoint001_Row01_Well01_Alexa488_zstack001_r003_c005.tif' │ ├── .size = 2333056 │ ├── .hash = '0aoXxT857VvKAGo9UQo-8g' │ ├── .path = s3://lamin-eu-central-1/r7YUayXjktSb/.lamindb/Md4OouMExlWS2YfZ0000.tif │ ├── .created_by = testuser1 (Test User1) │ ├── .created_at = 2025-03-07 13:51:25 │ └── .transform = 'Transfer from `scportrait/examples`' ├── Linked features │ └── FOV cat[ULabel] FOV1 │ cell_line_clone cat[ULabel] U2OS lcklip-mNeon mCherryLC3B clone 1 │ channel cat[ULabel] Alexa488 │ genotype cat[ULabel] WT │ imaged structure cat[ULabel] LckLip-mNeon │ magnification cat[ULabel] 20X │ microscope cat[ULabel] Opera Phenix │ stimulation cat[ULabel] 14h Torin-1 │ study cat[ULabel] autophagy imaging │ resolution float 0.597976081 └── Labels └── .cell_lines bionty.CellLine U-2 OS cell, U2OS .ulabels ULabel WT, 14h Torin-1, U2OS lcklip-mNeon mCher…
In addition, we create a Collection
to hold all Artifact
that belong to this specific imaging study.
collection = ln.Collection(
artifacts,
key="Annotated autophagy imaging raw images",
description="annotated microscopy images of cells stained for autophagy markers",
).save()
Let’s look at some example images where we match images from the same clone, stimulation condition, and FOV to ensure correct channel alignment.
FOV_example_images = (
metadata_files.sort_values(by=["cell_line_clone", "stimulation", "FOV"])
.head(3)
.reset_index(drop=True)
)
fig, axs = plt.subplots(1, 3, figsize=(15, 5))
for idx, row in FOV_example_images.iterrows():
path = ln.Artifact.using("scportrait/examples").get(key=row["image_path"]).cache()
image = imread(path)
axs[idx].imshow(image)
axs[idx].set_title(f"{row['imaged structure']}")
axs[idx].axis("off")
FOV_example_images = (
metadata_files.sort_values(by=["cell_line_clone", "stimulation", "FOV"])
.tail(3)
.reset_index(drop=True)
)
fig, axs = plt.subplots(1, 3, figsize=(15, 5))
for idx, row in FOV_example_images.iterrows():
path = ln.Artifact.using("scportrait/examples").get(key=row["image_path"]).cache()
image = imread(path)
axs[idx].imshow(image)
axs[idx].set_title(f"{row['imaged structure']}")
axs[idx].axis("off")
→ mapped records: Artifact(uid='jVytS8AyAHmHkYR30000'), CellLine(uid='4oLoqaUP'), ULabel(uid='Aj8KGwbh'), ULabel(uid='A2945i5P'), ULabel(uid='JIbWVXma'), ULabel(uid='XKI9Mx33'), ULabel(uid='CrR7fgIZ'), ULabel(uid='QrU6fxsG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ mapped records: Artifact(uid='cw4F6bUB9zuMthCY0000'), CellLine(uid='4oLoqaUP'), ULabel(uid='HK8F1lVS'), ULabel(uid='Aj8KGwbh'), ULabel(uid='A2945i5P'), ULabel(uid='JIbWVXma'), ULabel(uid='CrR7fgIZ'), ULabel(uid='xhpmj7p7'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ mapped records: Artifact(uid='7TZGXvbA0JLL68hR0000'), CellLine(uid='4oLoqaUP'), ULabel(uid='Aj8KGwbh'), ULabel(uid='A2945i5P'), ULabel(uid='JIbWVXma'), ULabel(uid='AyafKoQw'), ULabel(uid='CrR7fgIZ'), ULabel(uid='xHqZKcIG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ mapped records: Artifact(uid='Ov8FnKzHMNY0XVJa0000'), CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='EgihgpJC'), ULabel(uid='GadMJgvN'), ULabel(uid='XKI9Mx33'), ULabel(uid='1KPobNqK'), ULabel(uid='QrU6fxsG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ mapped records: Artifact(uid='Lwk8shsYe0V5bMgd0000'), CellLine(uid='4oLoqaUP'), ULabel(uid='HK8F1lVS'), ULabel(uid='9hHptuyb'), ULabel(uid='EgihgpJC'), ULabel(uid='GadMJgvN'), ULabel(uid='1KPobNqK'), ULabel(uid='xhpmj7p7'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
→ mapped records: Artifact(uid='YuyVn060M4FxATPz0000'), CellLine(uid='4oLoqaUP'), ULabel(uid='9hHptuyb'), ULabel(uid='EgihgpJC'), ULabel(uid='GadMJgvN'), ULabel(uid='AyafKoQw'), ULabel(uid='1KPobNqK'), ULabel(uid='xHqZKcIG'), ULabel(uid='PKiCEP1h'), ULabel(uid='HRRTqARL'), ULabel(uid='e82fx2wm')
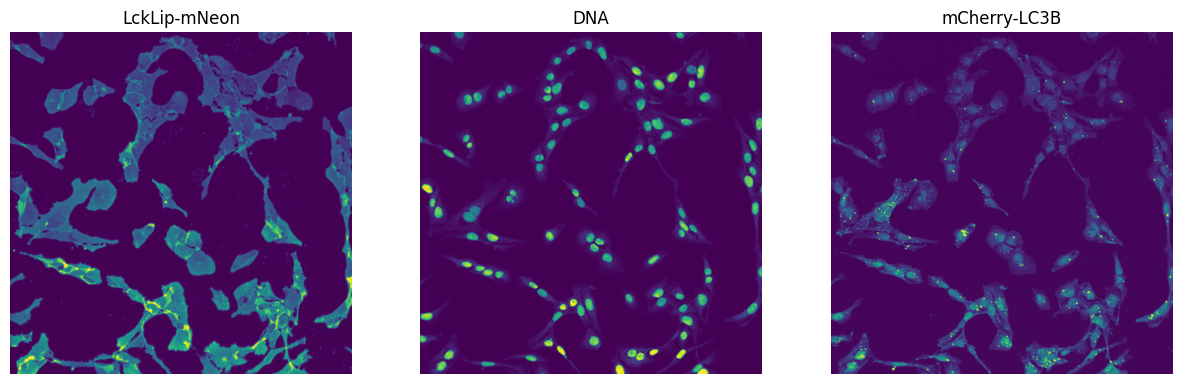
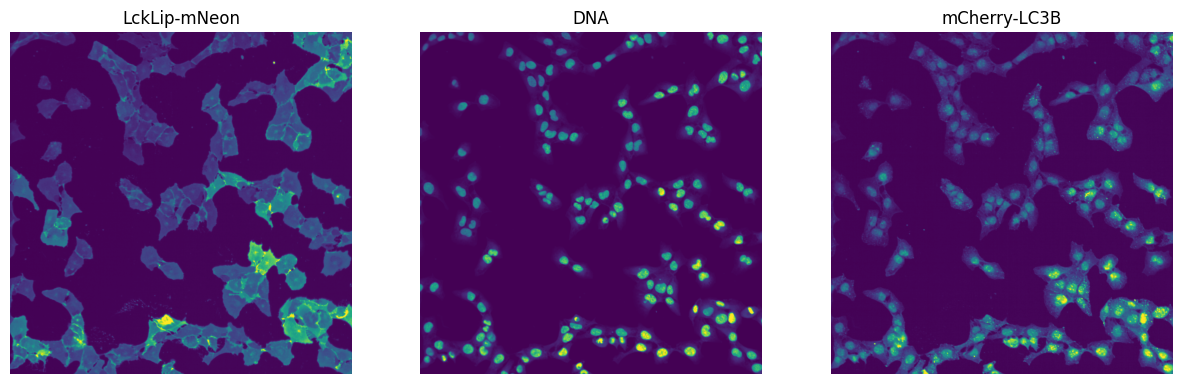
ln.finish()
Show code cell output
→ finished Run('4TQVxxtn') after 3m at 2025-06-03 16:34:29 UTC